In this post we will cover everything you need to know about React Fragments in a way that everyone will be able to understand, take away and use in your React applications.
A React Fragment is a useful tool when it comes to building React applications as fragments make it simple and easy to group components and elements together without polluting the DOM by adding in parent elements just for grouping purposes.
By the end of this post you will be able to confidently make use of fragments and know everything you will ever need to know about them.
What is a React Fragment?
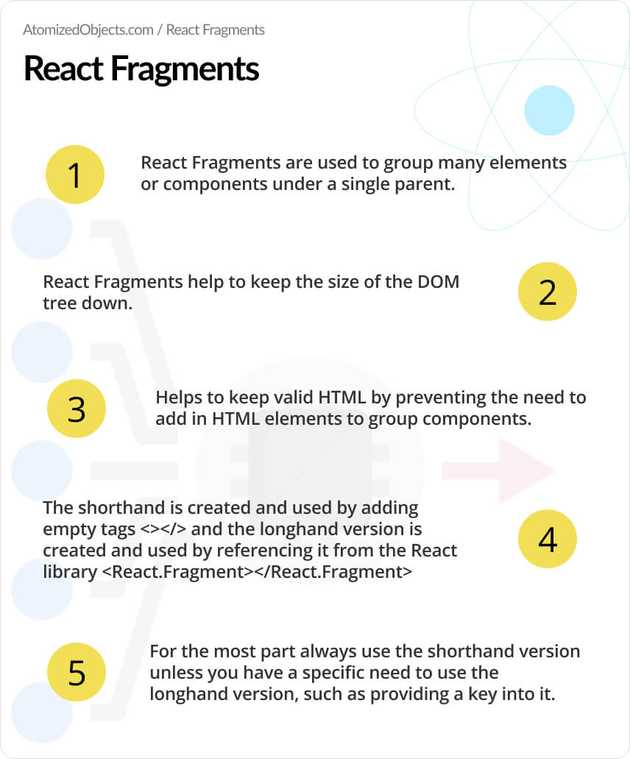
A React Fragment is an empty React Element that can be used to group elements and components together in React to keep the validity of HTML and without adding the additional noise of itself to the end result of the DOM.
What that means is that the React Fragment won’t show up in the DOM and it will act like it is not there, but it enables you to group your components together and return them even when that parent wrapping DOM node is required by React.
Here is a quick visual example of how this looks in practice with a React Fragment:
<React.Fragment>
<p>Hello World</p>
<p>This is a React Fragment example</p>
</React.Fragment>
The above code would be rendered into the following HTML:
<p>Hello World</p>
<p>This is a React Fragment example</p>
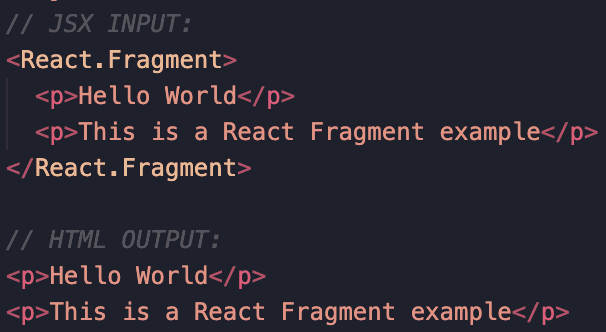
As you can see we grouped the elements together in our JSX but that stays only within the JSX and does not make its way into the DOM/HTML of the application once rendered which helps to keep valid HTML and reduce the overall size of the DOM tree.
Why are React Fragments needed?
At this point you might be wondering why React Fragments are really even needed, and what problems they solve.
When we create components in React there are times where we need to return multiple components such as with lists or groups of items.
Here is an example of a component that returns multiple items:
<ul>
<FruitListItems />
</ul>
In this situation if we just return the list items in FruitListItems
with no parent element then React will throw an error saying something along the lines of: JSX expressions must have one parent element
.
const FruitListItems = () => (
<li>Apple</li>
<li>Orange</li>
<li>Grape</li>
<li>Pear</li>
)
// JSX expressions must have one parent element
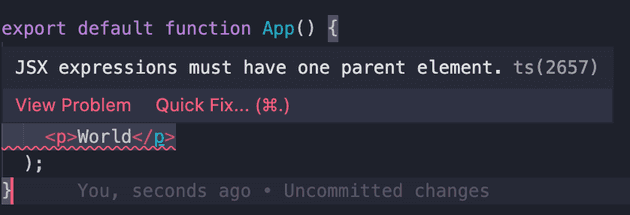
Now, in this same situation it would not be valid to return a different html element as the parent because the list items need to be direct children of the list element so we can’t just return any html element such as a div as the parent in order to group them together.
This is where React Fragments come in handy so that we can group them together under a single parent so that it satisfies React as well as satisfying the validity of the HTML by not creating elements in places that shouldn’t exist.
Here is how this would look:
const FruitListItems = () => (
<React.Fragment>
<li>Apple</li>
<li>Orange</li>
<li>Grape</li>
<li>Pear</li>
</React.Fragment>
);
Which would remove any errors and then output the following HTML:
<ul>
<li>Apple</li>
<li>Orange</li>
<li>Grape</li>
<li>Pear</li>
</ul>
This is why React Fragments are needed, but there is another use case where the HTML would be valid with or without an additional parent element and in these cases by using a React Fragment it helps to keep the overall size of the HTML DOM tree down which helps with performance.
It probably won’t have a large effect if it is only done in one or two places but if used over a large application it can really save on the overall size of the DOM tree.
How to use a React Fragment
Thankfully using a React Fragment is nice and easy to do and we are provided with two different options from React.
We have the longhand version <React.Fragment>
and the shorthand version <>
. For more information on the differences between the two I highly recommend having a read through What are the differences between React.Fragment vs <>
.
However, we will go over this a little here later on in this post as well.
For now let’s take a look at how you can use both the longhand and shorthand versions of React Fragment.
Here is an example of the longhand version of a React Fragment:
import React from "react";
export const FruitListItems = () => (
<React.Fragment>
<li>Apple</li>
<li>Orange</li>
<li>Grape</li>
<li>Pear</li>
</React.Fragment>
);
As you can see in the above example we have imported React
into this snippet because in order to access the longhand version of a React Fragment we need to first get it from the React library.
Once we have imported it we just need to wrap our elements in the Fragment by treating the fragment just like you would with any other component in JSX with an opening and closing tag encapsulating the child elements.
<React.Fragment>{children}</React.Fragment>
Once we have done that, we now have a working fragment in our code and there is nothing more to do.
Moving onto the shorthand version of a React Fragment, it is used in the exact same way except that you don’t need to reference the React library to make use of it and React will handle this behind the scenes for us.
It is worth noting at this point that depending on which version of React you are using, you might still need to import React into your file to be able to create components with JSX.
Let’s take a look at the same example but this time with the shorthand version of a React Fragment:
export const FruitListItems = () => (
<>
<li>Apple</li>
<li>Orange</li>
<li>Grape</li>
<li>Pear</li>
</>
);
As you can see it is much shorter and much simpler to include into the component when using the shorthand version of React Fragments.
All we need to do is wrap our elements in empty opening and closing tags <>{children}</>
and React will understand that they are fragments and will remove them from the output.
For the most part you will want to make use of the shorthand version because it is faster to type and you don’t have to worry about importing it or referencing it from the React library.
The only time that you might need to use the longhand version is when you need to include an attribute such as a React Key</> when rendering from lists.
And in this case once again it is the same as adding in props or attributes to any other component or element.
Here is an example of it:
{
someArray.map(({ copy1, copy2, id }) => (
<React.Fragment key={id}>
<p>{copy1}</p>
<p>{copy2}</p>
</React.Fragment>
));
}
You can read more about keys here in this in depth guide.
However, the reason why you need to use the longhand version of a React Fragment is because the shorthand version does not support attributes, but the longhand version does.
React Fragment vs div
The main difference between a React Fragment and a div is that the div will be rendered into the DOM and the React Fragment won’t be rendered into the DOM.
This will mean that by using a fragment wherever possible it will not only save on DOM size but it will enable you to keep valid HTML even if you need to return a group of elements to a parent list or table and so on.
React Fragments do have their limitations however, so if you need to style your parent container or add any attributes (except from a key), event listeners or any props you will need to use a HTML element rather than a fragment.
For the most part in these cases a div tag will be used but you can equally use any other HTML element as long as it fits into your HTML to create a valid DOM tree.
You can read more about this in detail on React Fragment vs Div.
Using the key prop with a React Fragment
The key prop in React is not something unique to React Fragments but it might be noticed more with them because you need to make use of the longhand version of React Fragments when using the key prop because the shorthand version of react fragments does not support props or attributes.
But the key prop itself is something that can be passed into any element or component within React as long as it supports attributes.
With this in mind we won’t go into too much depth because it is not so closely related to fragments.
But to summarize it, the key prop is a prop that requires a unique field passed into it whenever you are rendering components based off of an array.
The uniqueness only needs to be unique within the given array and not global to the React app.
React uses the key to determine what has changed within an array for each render so it knows how to update the DOM properly, and if you don’t provide a key prop it could cause some edge cases and bugs depending on your array and what you are using it for.
The reason why it is often coupled with a react fragment is based on what is happening.
Usually when a list is being rendered into elements or components, you might need to create multiple elements/components rather than just a single one.
Because of this we fall back to the same reason why fragments are needed within React in the first place which is to group these components and elements together under a single parent element.
When we do this the key always needs to be applied to the root element in the newly created component which will usually be a fragment or an element such as a div.
Here is an example of how this looks:
import React from "react";
const fruit = ["Apple", "Orange", "Grape", "Pear"];
export const FruitListItems = () => (
<div>
{fruit.map((fruit, index) => (
<React.Fragment key={fruit}>
<p>{fruit}</p>
<p>index: {index}</p>
</React.Fragment>
))}
</div>
);
As you can see in the above example we are using the “fruit” as the key because it is always unique within that array, and where we are creating a new component from array.prototype.map for each “fruit” with multiple elements we need to wrap in in a fragment to have a single parent element.
And because we need to include a key we are therefore having to use the longhand version of a React Fragment.
React fragment longhand vs React Fragment shorthand
The core difference between the longhand and shorthand React Fragment is that the shorthand does not support attributes whereas the longhand does.
The shorthand version was introduced in React v16.2.0.
The only attribute you are likely to ever use with a Fragment is a key like we have talked about above, so as long as the key is not needed then it will be quicker and easier to make use of the shorthand.
You can read more about this in detail on React.Fragment
vs<>
.
Summary
There we have React Fragments, if you want more like this be sure to check out some of my other posts!