This will be a short post that looks at all the differences and similarities between React.Fragment vs <>.
After reading this post you will be able to know exactly when to use one or the other form of fragments in all your React applications.
What are the differences between React.Fragment vs <>?
While both React.Fragment vs <> let you group lists of components, the main difference between React.Fragment and <> is that the <> is simply a shorthand version of writing out React.Fragment that does not support attributes.
In other words <> is quick and easy but you can’t add attributes to it, and React.Fragment takes slightly longer to type out but will enable you to include attributes such as the key field.
Here is an example of both being used:
import React from 'react';
// shorthand with <>
function Info() {
return (
<>
<h1>Hello World</h1>
<p>This is an example of a shorthand fragment using {"<>"}</p>
</>
);
}
// longhand with React.Fragment
function InfoLonghand() {
return (
<React.Fragment>
<h1>Hello World</h1>
<p>
This is an example of a longhand fragment using {"<React.Fragment>"}
</p>
</React.Fragment>
);
}
What this means is that the shorthand fragment (<>
) is perfect if you just need to group multiple components or elements together in a parent and you don’t want to create additional HTML DOM elements.
The React.Fragment version can be used in exactly the same way except that you are also able to add attributes such as the key
attribute to it which is often needed when looping over arrays to create lists of components.
When to use React.Fragment vs <>?
It is safe to say that unless you have a specific need to use React.Fragment, then you should always use the shorthand version (<>
) because it is much simpler, easier and cleaner to use.
The main use case for when you might want to use the longhand version, React.Fragment, is when mapping an array into components in your JSX so that you can pass a key to the React.Fragment.
When you do this you will need to pass in a key attribute so that React can track the order between renders.
Here is an example of this:
import React from 'react';
function Info() {
const fruits = ["apples", "bananas", "grapes"];
return (
<div>
{fruits.map(fruit => (
<React.Fragment key={fruit}>
<p>Our next fruit is…</p>
<p>{fruit}!</p>
</React.Fragment>
))}
</div>
);
}
Why do we need to use fragments in React?
In React fragments are needed because you cannot return a list of components as a component, there always needs to be a parent element or component.
If you have ever attempted to return a list of components without a parent you will see an error such as “JSX expressions must have one parent element”.
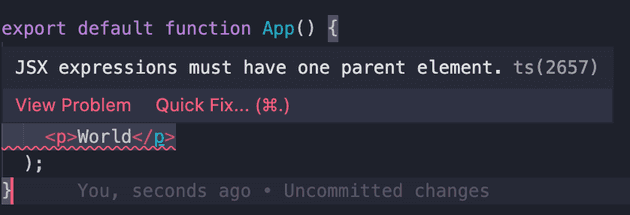
There are a few ways to solve this and one of them is to just wrap everything in another HTML element such as a div.
The problem with this approach (and the birth of fragments) is that we are adding needless HTML elements to the DOM which is creating a larger DOM tree which is not ideal for users and can lead to a number of problems.
Instead, we can make use of fragments which do the same thing but it avoids creating an extra HTML element.
So we create a parent for React but we don’t create a needless HTML element for the user.
Summary
It is quickly worth noting that this is exactly the same in React native as well.
There we have what are the differences between React.Fragment vs <>, if you want more like this be sure to check out some of my other posts!