In React you can’t return a group of components, there always has to be a root component or element in order to be able to return JSX from a component.
In this article we will look at React.Fragment vs div so that you know the right kind of root element to use to wrap your group of components together.
What is the difference between React.Fragment vs div?
The main difference between React.Fragment vs div is that using a React.Fragment will not add any additional elements into the DOM tree, whereas, using a div will add a div to the DOM tree.
This is fine if you need to have a div in the DOM tree but if you don’t and you are just using it as a way to return multiple components in React then this is where Fragments come in handy.
Let’s take a look at an example input component in JSX:
export default function App() {
return (
<p>Hello</p>
<p>World</p>
)
} // By not wrapping you will get the following error: "JSX expressions must have one parent element"
Currently trying to use this would result in the following error: "JSX expressions must have one parent element"
.
To solve this problem we are going to look at an example with a div and a React.Fragment and then compare the output of both.
Here is our input example using a div:
export default function App() {
return (
<div>
<p>Hello</p>
<p>World</p>
</div>
);
}
And here is our input example using a fragment:
export default function App() {
return (
<React.Fragment>
<p>Hello</p>
<p>World</p>
</React.Fragment>
);
}
(You can actually shorten <React.Fragment></React.Fragment>
to <></>
which is the shorthand version, I have a post on the differences here)
As you can see the only difference in our two inputs is that one uses a div and the other uses a fragment.
Let’s now take a look at the outputted HTML to see the differences:
Here is the output HTML example using the div input:
<div>
<p>Hello</p>
<p>World</p>
</div>
And the output with the fragment input:
<p>Hello</p>
<p>World</p>
As you can see by using the fragment we have been able to remove the additional div element from our DOM tree.
Whilst this is only a small difference here, when looked at across an entire React application it can make a big difference.
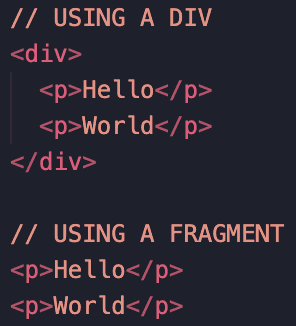
When to use a React.Fragment over a div
For the most part it is safe to assume that you will always want to use a React.Fragment over a div wherever possible in order to keep the size of the DOM down.
The problem is, it is not always possible to use a React.Fragment in place of a div element because there might be certain things that you will need the wrapping element to have which we will get into in the next section.
But for the most part if you just need to group some components or elements together in a React application then React.Fragment is almost always the better choice to reduce the overall DOM size.
When to use a div over a React.Fragment
As mentioned in the above section, sometimes you can’t always use a react fragment in place of a div because there might be certain things you need the wrapping element to have.
And these are the times when you will want to make use of a div (or any other relevant html element) over a React.Fragment.
There might be many edge cases to this but some of the main situations that this might occur is when you need to apply styling, add event handlers or add attributes to the root element.
Where a React.Fragment does not get rendered into the DOM it can’t support these things and you will therefore have to make use of an actual html element such as a div.
Once again there is an exception to this which is when it comes to React keys.
A React Key is just a way that React can track components in lists across renders to prevent strange behavior (read more about keys here).
One of the few attributes/props that a React.Fragment will support is the key prop.
This comes in handy when you are looping over data and creating components off of them so that you can then avoid creating additional HTML DOM elements for each one and you can just provide your key to the React.Fragment directly instead.
Summary
There we have react.Fragment vs div, if you want more like this be sure to check out some of my other posts!
Another article that might interest you is one I have recently written about React.Fragment vs <>, be sure to check it out.