This post will cover the JavaScript forEach method (Array.prototype.forEach) in detail without too much technical jargon so you will have everything you need right here without having to look any further!
JavaScript forEach (Array.prototype.forEach)
The JavaScript forEach method (Array.prototype.forEach) is a function that iterates through an array and will execute a callback that is provided to it on each iteration.
The forEach method was added in ECMAScript 5 specification and has full browser and node.js support.
The callback that is provided will be passed a value, an index and an array as arguments.
For a quick example before we carry on, given you have an array of numbers 1-4 [1,2,3,4]
you will then be able to call .forEach
on that array and pass in the callback function (value, index, arr) => { ... }
, which will then be called on each iteration with the value, the index and the entire array as arguments.
Here is how the above example will look as JavaScript code:
[1, 2, 3, 4].forEach((value, index, fullArray) => {
console.log(value, index, fullArray); // "1, 0, [1,2,3,4]", "2, 1, [1,2,3,4]", ...
});
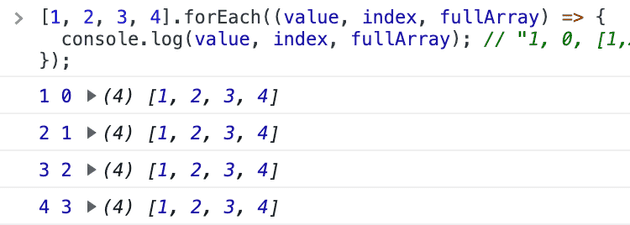
This works because the forEach method has been added to the Array’s prototype chain which all Array objects in JavaScript inherit from provided you are using a version that supports it (almost all browsers and node.js versions do support Array.prototype.forEach).
The main use cases are where you need to perform a for loop over an array that you do not need to save the value from.
Most coding practices that make use of JavaScript prefer the use of the JavaScript forEach method over the more traditional for loop, or while loops because it is simpler and more compact to implement.
It is important to note however that Array.prototype.forEach is not a direct replacement for for or while loops and there are some key differences between the two.
While you are able to get the same values from a forEach loop as you would a for loop, the way to break or continue are different.
In fact in a JavaScript forEach loop you will be unable to use break or continue statements because they are only for use in traditional loops and cannot be used in functions.
We will go into more detail about this under the sections JavaScript forEach continue, and JavaScript forEach break later on in the post.
Array.prototype.forEach parameters and syntax
Array.prototype.forEach has two parameters that you can pass in, the first accepts a callback function and second accepts an argument that will be bound to the this keyword.
The callback function that you can pass in can accept three parameters which will be the value of the current iteration, the index of the current iteration and lastly the entire array.
[].forEach(callbackFn, thisArg);
// callback function accepting the value only
[].forEach((value) => { ... })
// callback function accepting the value and index
[].forEach((value, index) => { ... })
// callback function accepting the value, the index and the fullArray
[].forEach((value, index, fullArray) => { ... })
// callback function accepting the value, the index and the fullArray, with thisArg
[].forEach((value, index, fullArray) => { ... }, thisArg)
The callbackFn
is a function that you can provide that accepts the following arguments:
value
is the value of the current iteration.index
is the index of the current iteration.fullArray
is the array you are iterating through.
The thisArg
is a value that can be passed in that will then be used as the this
keyword for your callback function.
For almost all use cases you will only ever need to pass in the callback function and most of the time you will only need to accept the value and the index.
The fullArray argument can be handy though, especially when you need to make use of or create algorithms that compare previous entries or check for duplicates.
For example here is an algorithm that will use the JavaScript forEach method to check for any duplicated numbers in an array:
const countDuplicates = arr => {
let duplicateCount = 0;
arr.forEach((value, index, fullArr) => {
if (index === 0) return;
if (value === fullArr[index - 1]) {
duplicateCount += 1;
}
});
return duplicateCount;
};
countDuplicates([1, 2, 3, 3, 4]); // 1
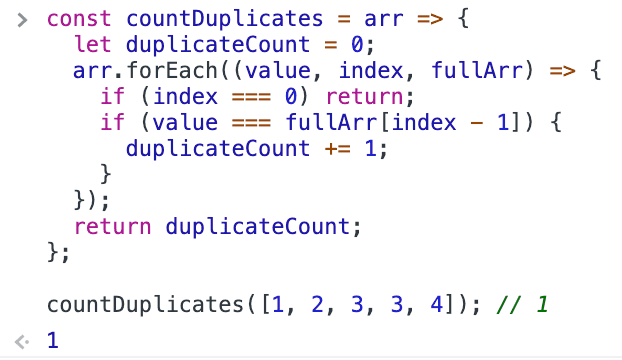
Array.prototype.forEach return value
The return value for a successful execution of the Array.prototype.forEach method is undefined
.
The forEach method does not return any values from the iterations because it does not modify the original array and therefore will have no effect on it and does not need to return anything.
Space and Time Complexity of JavaScript forEach (Big O Notation)
The Space and Time Complexity (Big O) for Array.prototype.forEach is at the best case and average case of O(n)
because there is a single loop performed.
It is worth noting that this is entirely dependent on the implementation of it.
Array.prototype.forEach Polyfill
Many polyfills exist for the JavaScript forEach method, and whilst you probably won’t need a polyfill because it has full browser and device support, you can find a polyfill in the core-js package, here on the MDN docs, or you can even create your own simplified polyfill for your own needs like so:
if (!Array.prototype["forEach"]) {
Array.prototype.forEach = function (callbackFn) {
if (!this) {
throw new Error(
"Array.prototype.forEach must be called on a valid array"
);
}
var i = 0;
while (i < this.length) {
callback(this[i], i, this);
i++;
}
};
}
It is worth noting that the above example is not a direct polyfill and is an example of creating a custom polyfill to emulate a basic version of the forEach method for your own specific needs.
For a more accurate polyfill take a look at adding core-js to your project or seeing the example on MDN.
With all this said, you should not need to find a polyfill because most devices have support for the forEach method, and if they do not, you can make use of the more traditional for or while loop to produce the same results.
Array.prototype.forEach Browser Support
Array.prototype.forEach is supported across all major devices and browsers and is considered safe to use in most production environments.
This includes:
- Google Chrome
- Edge
- Firefox
- Safari
- IE
- Opera
- Android
- IOS
- Samsung
- Node.Js
- Deno
For more information about browser compatibility see can I use and the MDN docs.
JavaScript forEach index
Just like in the examples above the JavaScript forEach index is the second argument passed into the callback you provide to the forEach method.
The index works in the same way as any other loop in JavaScript.
Here is a quick example of using the index in the JavaScript forEach loop:
// find index of letter 'b' using the forEach loop
let indexOfB = -1;
["a", "b", "c"].forEach((value, index) => {
if (value === "b") {
indexOfB = index;
}
});
console.log(indexOfB); // 1
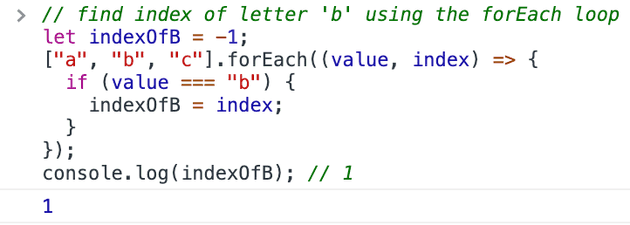
For more information on how you can use the JavaScript forEach index see the full post on it here.
JavaScript forEach object
It is possible to use the Array.prototype.forEach method with an object, but we first need to convert an object into an array of key value pairs before we will be able to iterate through it.
We can convert an object into an array in a few ways but the most common ways are to use either Object.entries, or Object.keys.
Both of these methods will accept an object and return it as an array.
If the Object.keys
method is used then it will return an array of the object’s keys that can then be used to reference the values via a lookup operation.
Alternatively if Object.entries
is used then it will return an array of key value pairs so no additional lookup is needed.
Here is an example of using JavaScript forEach with Object.entries:
const example = { hello: "world", number: 8 };
// the [key, value] argument here is just destructuring the value that is provided from forEach.
Object.entries(example).forEach(([key, value], index, fullArray) => {
console.log(key, value, index, fullArray); // "hello, world, 0, [['hello', 'world'], 'number', 8]", ...
});
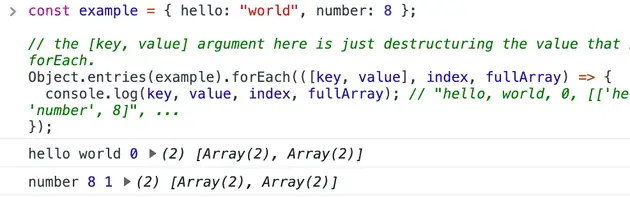
And here is the same example but using JavaScript forEach with Object.keys instead:
const example = { hello: "world", number: 8 };
Object.keys(example).forEach((key, index, fullArray) => {
const value = example[key];
console.log(key, value, index, fullArray); // "hello, world, 0, ['hello', 'number']", ...
});
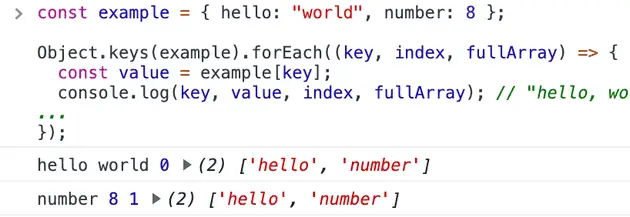
For more information on how you can use the JavaScript forEach method with an object see the full post on it here.
JavaScript forEach break
The JavaScript forEach method can’t use the break keyword, and can’t terminate early like you can with a for loop or while loop.
If you need to use a break then Array.prototype.forEach is not the right method to use here, it would be better to use an alternative such as a for loop, while loop, or Array.prototype.some.
It is possible to stop future iterations in a forEach loop by throwing an exception, but this would be considered a “hack” because that is not the intended use for it.
For more information on using break in a JavaScript forEach loop see my full post on it here.
JavaScript forEach continue
Similarly to the break statement, you can’t use a continue statement within a JavaScript forEach loop either.
With that said, it is possible to “continue” in a forEach by using the return statement instead.
By returning from the function you are then finishing the blocking code so the loop can move onto the next iteration so it emulates something similar to using the continue statement in a for loop.
Here is an example of continuing in JavaScript forEach loop:
[1, 2, 3, 4, 5].forEach((value, index) => {
if (index > 2) return;
console.log(value); //1, 2, 3
});
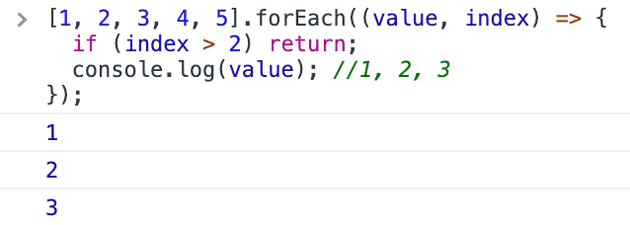
For more information on how to continue in a JavaScript forEach loop see the full post on it here.
JavaScript forEach async
Array.prototype.forEach will not wait for promises to complete which means you can’t use async callbacks or any other form of promises for the callback function.
Instead it would be better to use an alternative such as a for loop to ensure than your promises do complete before moving into the next iteration.
For more information on JavaScript forEach async see my full post on it here.
JavaScript forEach examples
Here are a few JavaScript forEach examples to reference if you ever need a quick solution.
Printing values
[1, 2, 3].forEach((value, index) => {
console.log(`iteration value: ${value}, iteration index: ${index}`);
});
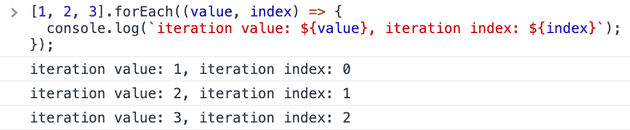
For loop vs forEach loop comparison
// JavaScript forEach loop
[1, 2, 3].forEach((value, index) => {
console.log(`iteration value: ${value}, iteration index: ${index}`);
});
// equivalent JavaScript for loop
const arr = [1, 2, 3];
for (let i = 0; i < arr.length; i += 1) {
const value = arr[i];
console.log(`iteration value: ${value}, iteration index: ${i}`);
}
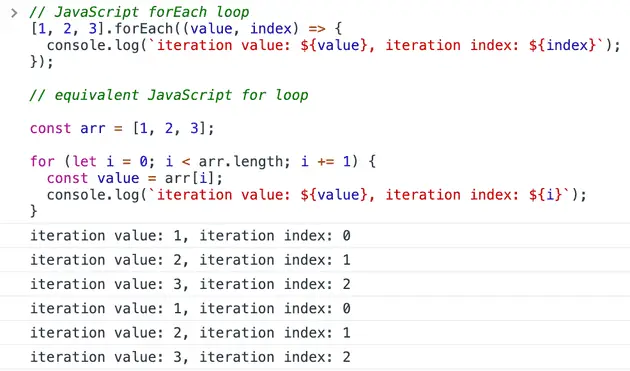
JavaScript forEach continue example
[1, 2, 3, 4, 5].forEach((value, index) => {
if (index < 2) return;
console.log(value); // 4, 5
});
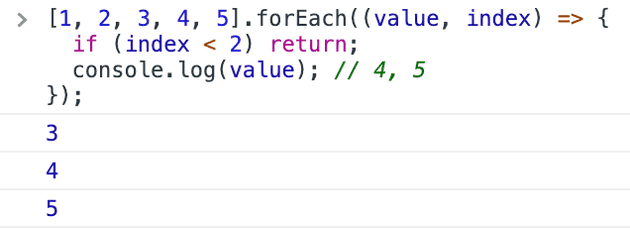
Finding duplicate elements in position
let duplicateCount = 0;
[1, 2, 2, 3, 4, 4].forEach((value, index, fullArr) => {
if (index === 0) return;
if (value === fullArr[index - 1]) {
duplicateCount += 1;
}
});
console.log(`${duplicateCount} duplicates found in array`);
// 2 duplicates found in array
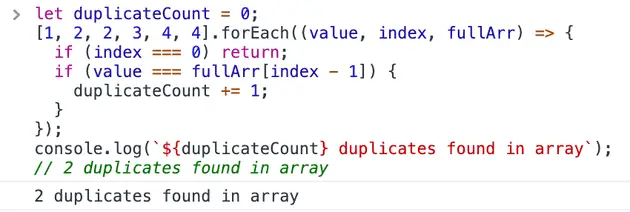
To find duplicates regardless of the position, try sorting the array first.
For more examples of the JavaScript forEach loop, click here.
JavaScript forEach alternatives
The alternatives to the Array.prototype.forEach method in JavaScript is really anything that can iterate over an array.
Some direct alternatives:
- JavaScript for loop
- JavaScript while loop
Other alternatives that provide slightly different results to the JavaScript forEach method and have slightly different use cases:
- Array.prototype.map - used to create a new array from an existing array.
- Array.prototype.some - iterate until you find what you are looking for.
- Array.prototype.filter - used to create a new array whilst filtering out any unwanted values.
Other JavaScript forEach resources
For more resources and information about the JavaScript forEach method I recommend taking a look at the following:
- MDN Documentation
- ECMA Documentation
- How to get the index in a forEach loop
- How to use forEach with an object in JavaScript
- How to break a JavaScript forEach loop
- How to continue in a JavaScript forEach loop
- Is the JavaScript forEach method async?
- How to get the JavaScript forEach key value pairs from an object
- JavaScript forEach examples
Summary
There we have the JavaScript forEach(Array.prototype.forEach) method, if you want more like this be sure to check out some of my other posts!