In this post we will be covering how to use forEach with an Object in JavaScript with as little technical jargon as possible so you will have everything you need right here without having to look any further!
It is possible to use Array.prototype.forEach with an object in JavaScript and that is exactly what we are going to look at doing in this post.
The forEach method is a method attached to the Array object and is not an object method so to be able to use it with an object we are going to need to do some extra work to be able to perform a conversion of object to array in JavaScript first.
If you want to find how to get the index in a forEach loop in JavaScript, I have a post here that shows how to in detail.
Let’s get started and look at how we can use forEach Object in JavaScript.
How to use forEach with an Object in JavaScript
In as few words as possible, to use forEach with an Object in JavaScript we need to convert the object into an array.
The best method to do this is by using Object.entries because the entries method will return the object properties as key value pairs in the form of an array.
When we combine this with the forEach method it means we can then effectively use forEach with an Object for iterating through the keys and values of an object.
Here is an example of iterating in javascript foreach object with key value pairs:
const myObject = {
someKey: "some value",
hello: "World",
js: "javascript foreach object",
}
Object.entries(myObject).forEach(([key, value]) => {
console.log(key, value) // "someKey" "some value", "hello" "world", "js javascript foreach object"
})
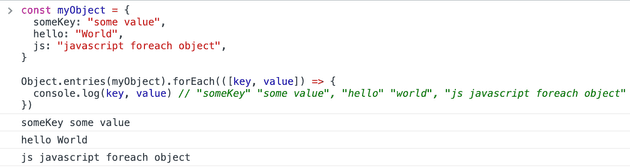
As you can see we have already been able to make use of forEach to be able to iterate through the properties of an object by first converting it into an array using Object.entries and then getting the key value pairs from it.
In the above example of Object.entries with forEach you will notice that the argument that we are accepting is [key, value]
that is because we are destructuring an array.
When using Object.entries, it will convert the object into an array of key value pairs which are stored as nested arrays with a total of 2 array items in each, the key and the value.
The first index in one of these nested arrays will be the key and the second index will be the value.
So for example:
const objectEntries = [["someKey", "some value"]]
const firstEntry = objectEntries[0]
const key = firstEntry[0]
const value = firstEntry[1]
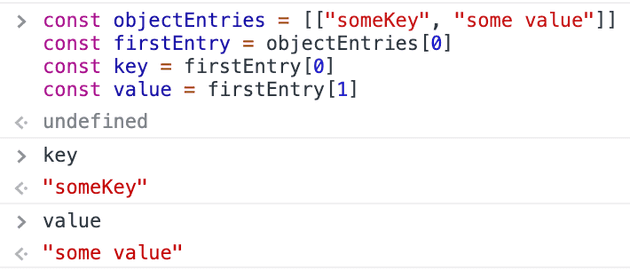
Whilst using entries with forEach with an object is the easiest way, there are still many other ways in which we can iterate through an object in JavaScript which could be more suited to your needs.
Using Object.keys with forEach in JavaScript
Another popular method for getting keys and values as an iterable from an object in JavaScript is with Object.keys and Array.prototype.forEach.
Using Object.keys will return all the keys of an object as an iterable array.
The reason why the Object.keys method works is because we can perform a lookup with each key in the array to find the value so that we end up with both the key and the value if needed.
Here is an example of how to use Object.keys with forEach to get the key value pairs:
const myObject = {
someKey: "some value",
hello: "World",
js: "javascript foreach object",
}
Object.keys(myObject).forEach(key => {
const value = myObject[key]
console.log(key, value) // "someKey" "some value", "hello" "world", "js javascript foreach object"
})
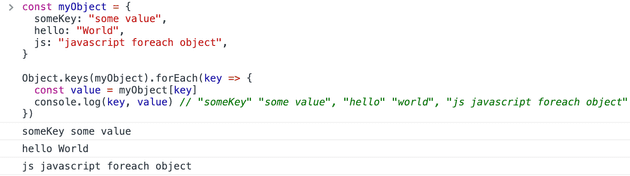
As you can see this method of using Object.keys with forEach works because we get an array of all the keys in the object that when we iterate through using forEach we can use each key to perform a lookup on the original object to then get the value from as well.
Using Object.values with forEach in JavaScript
The last way to convert an object into an array in JavaScript is to use the values in the object rather than key value pairs or the keys and we can do this with Object.values.
Using Object.values works in a similar way to Object.keys but instead of getting an array of the keys you get an array of the values from the object.
Using Object.values generally will have less use cases than the Object.entries or Object.keys because you can’t relate it to a key afterwards which means you can’t perform any further lookups.
With that said, if you just want to iterate through the values of an object using forEach then Object.values is perfect for it because it will provide all the values in a given object as an array that you can then loop through using Array.prototype.forEach.
Here is an example using Object.values with forEach in JavaScript:
const myObject = {
someKey: "some value",
hello: "World",
js: "javascript foreach object",
}
Object.values(myObject).forEach(value => {
console.log(value) // "some value", "world", "javascript foreach object"
})
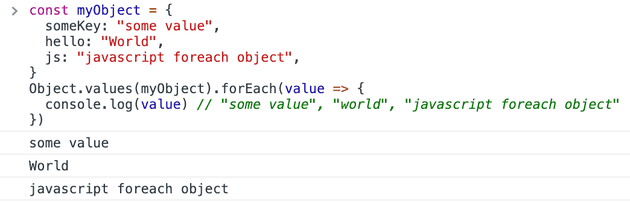
Creating your own JavaScript forEach object helper method
Now we have covered how to use forEach with an object by converting it to an array, let’s take a look at how we can improve on this and create our own helper method.
For the most part, you probably won’t need to create your own helper method because using Object.entries with Array.prototype.forEach is pretty concise and doesn’t add much overhead, but there might be the odd occasion where it is useful.
Here is an example of a generic helper method to run forEach on an object in JavaScript:
function forEachObject(someObj, callback) {
Object.entries(someObj).forEach((entry, ...rest) =>
callback(entry[0], entry[1], ...rest)
)
}
const myObject = {
someKey: "some value",
hello: "World",
js: "javascript foreach object",
}
forEachObject(myObject, (key, value) => console.log(key, value)) // "someKey" "some value", "hello" "world", "js javascript foreach object"
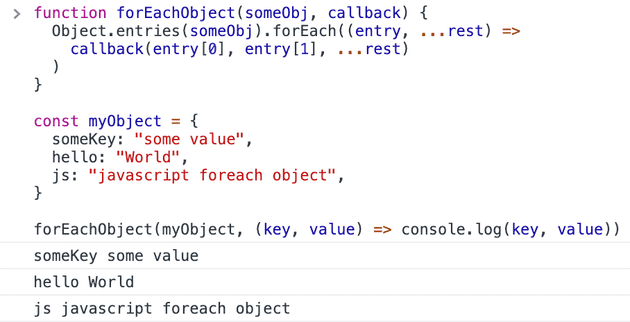
As you can see, creating a helper method to be able to convert an object to an array and then loop over it using forEach is pretty easy to do.
Summary
There we have how to use forEach with an Object in JavaScript, if you want more like this be sure to check out some of my other posts!