In this post we will be covering how to get the index in a forEach loop in JavaScript with as little technical jargon as possible so you will have everything you need right here without having to look any further!
In JavaScript we now have many ways to be able to loop/iterate through an array of items, such as for loops, while loops, for of loops, forEach, map and so on.
But, some of these methods make it a little harder to find the index when compared to a standard for loop.
This post will cover how to get the JavaScript forEach index whenever you use it.
Let’s get started.
How to get the index in a forEach loop in JavaScript
When you use the Array.prototype.forEach method you need to pass in a callback, your callback should then accept a set of arguments so you can deal with each array item however you need. The second argument passed into the callback you provide to the forEach method will be the current index the forEach loop is at, which is how you can get the index in a forEach loop.
The first argument of an Array.prototype.forEach loop will be the actual array item, the second argument will be the index, and the third argument will be the full array.
So to answer the question of how to get the index in a forEach loop in JavaScript, it is simply a case of accepting that second index argument in the callback function that gets passed into the forEach loop.
To visualize this let’s look at a JavaScript forEach index example:
const myArray = ["a", "b", "c", "d"]
myArray.forEach((arrayItem, index, fullArray) => {
console.log("The current array item is: ", arrayItem), "a", "b", "c", "d"
console.log("The current index is: ", index) // 0, 1, 2, 3
console.log("The full array is: ", fullArray) // ["a", "b", "c", "d"]
})
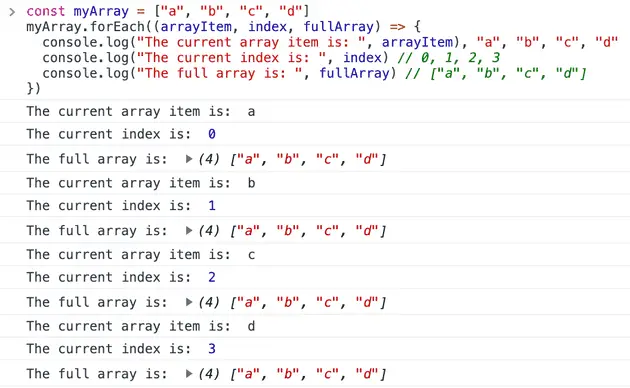
As you can see from this example of how to get the index in a forEach loop in JavaScript, we need to provide a callback function that accepts the index argument and then we can use it however we need.
The callback I have used for the forEach in this example is a simple arrow function which works really well with forEach loops because it means we don’t have to create a named function to pass in.
Using an arrow function in the forEach loop will save us time and save space in the code.
Breaking a forEach loop in JavaScript
If you need to break or stop a forEach loop, then it is probably the wrong method to be using because there is no way to stop it.
Instead a for loop might be better suited in comparison to a forEach loop, or if you need to use a method then you could use Array.prototype.some which will let you break out of the loop by returning true in your callback method.
Summary
There we have how to get the index in a forEach loop in JavaScript, if you want more like this be sure to check out some of my other posts!