In this post we will be covering how to break a JavaScript forEach loop with as little technical jargon as possible so you will have everything you need right here without having to look any further!
It is not possible to break a JavaScript forEach loop in the conventional sense, so what should you do instead?
In this post, we will cover everything you need to know about how to break a JavaScript forEach loop as well as the alternatives.
Let’s get started!
How to break a JavaScript foreach loop
If you need to to stop or perform a break in a JavaScript forEach loop then you probably shouldn’t be using a forEach loop, instead try using either a for loop, or Array.prototype.some which we will look at in more detail later in the post.
Array.prototype.forEach is designed to loop over every item in an array once whilst providing you with the array item, the index and the full array in a callback that you provide.
Because of this there is no way to just break out of it.
If you have tried to use a break statement within a forEach loop then you would have got the error “Uncaught SyntaxError: Illegal break statement”, which is expected because you can only use the break statement inside the direct scope of a loop, and not a function (even if the function is inside of the loop).
Here is an example of the error:
[1, 2, 3].forEach(a => {
console.log(a)
break
})
// Uncaught SyntaxError: Illegal break statement
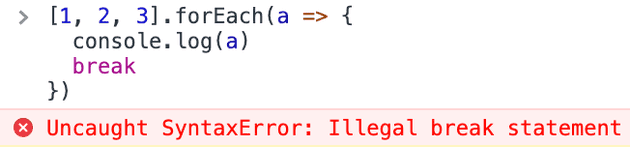
With all that said, we do have a few options for how to replicate a break in a JavaScript forEach loop if you still need to find a way to break a forEach loop.
How to break a JavaScript foreach loop using an exception
One option is to throw an exception with the callback you pass into the Array.prototype.forEach function.
By doing this you will cause an error which will stop all processes in your JavaScript application.
Because you don’t want your whole application to stop, we will need to wrap the Array.prototype.forEach loop in a try catch statement so we can catch the error that we are going to throw so that the error will act as a break statement.
Before we get into the example, this is really not ideal and as I have said at the start if you need to break out of a loop then your best option is to not use forEach and instead use a for loop to break, or the Array.prototype.some method.
Here is the example of how to use throwing an error to break a JavaScript foreach loop:
const breakFE = () => {
throw new Error("BREAK_FE")
}
const handleBreakFEError = e => {
if (e.message !== "BREAK_FE") {
throw e
} else {
console.log("javascript foreach break!")
}
}
try {
[1, 2, 3].forEach(item => {
if (item > 1) {
breakFE()
}
console.log(item)
})
} catch (e) {
handleBreakFEError(e)
}
// 1
// javascript foreach break!
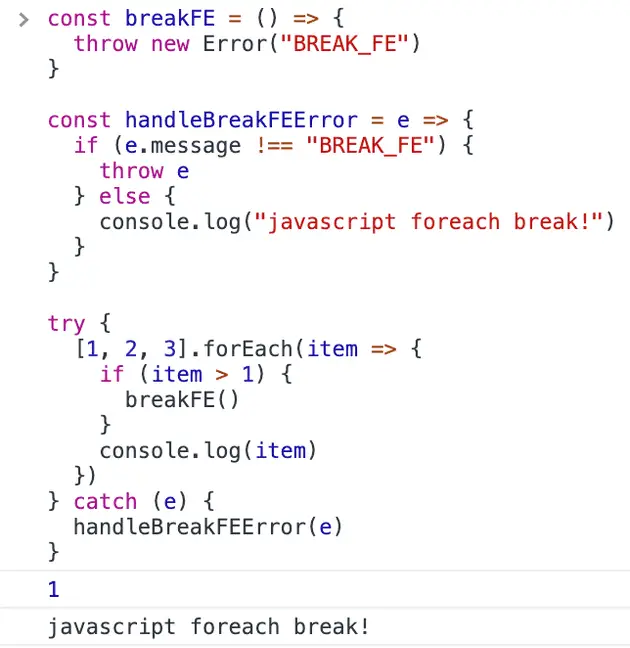
You could take this one step further and create a helper function, or even modify the Array object itself, but in general you should not do this and there is very little reason to do it.
Here is how that could look though:
Array.prototype.forEachWithBreak = function(callback) {
const breakFE = () => {
throw new Error("BREAK_FE")
}
const handleBreakFEError = e => {
if (e.message !== "BREAK_FE") {
throw e
} else {
console.log("javascript foreach break!")
}
}
try {
this.forEach((...args) => callback(...args, breakFE))
} catch (e) {
handleBreakFEError(e)
}
}
[1, 2, 3].forEachWithBreak((item, index, arr, breakFE) => {
console.log(item, index)
breakFE()
})
// 1 0
// javascript foreach break!
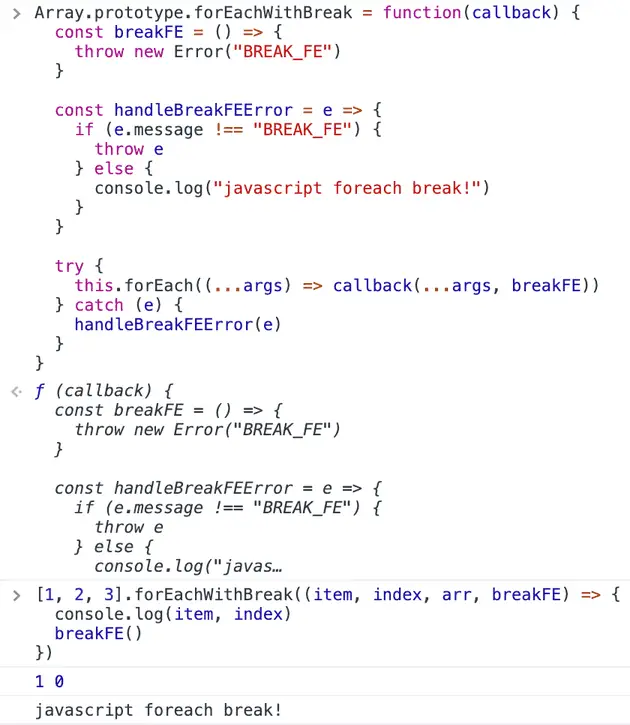
How to break a JavaScript foreach loop using an if statement and return
You can’t actually “break” a JavaScript forEach loop using an if statement because it will still perform all of the iterations on your array.
But you can drastically lower the amount of work that happens on each iteration.
If you are doing a large amount of computations on each iteration of your forEach loop then, whilst not perfect, you could use an if statement to mitigate doing that work which would be a little bit like calling continue on each iteration.
Here is an example of how you can use an if statement to skip computations in the forEach loop:
let breakFe = false;
[1, 2, 3].forEach(item => {
if (item > 1) {
return
}
console.log(item)
})
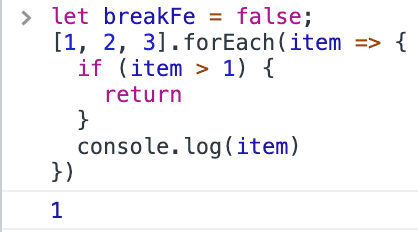
As you can see this works by just returning at the start of each iteration to keep the computational work as minimalist as possible by preventing any more code being run in your callback.
This is far from ideal, and it would be better to use a method that can enable you to break out or to use a for loop.
Alternatives to a JavaScript forEach break
There are a few options we can use for alternatives for the foreach loop in JavaScript, and they are the best solutions if you need to break out of a forEach loop.
Using a for loop as an alternative to a forEach loop to break
The first one, and the one I personally would recommend in this situation, would be to go with a standard for loop and break statement.
I have a full post on how you can break out of a for loop here.
Here is an example of how you can use a for loop to break a loop:
const myArray = [1, 2, 3];
for (let i = 0; i < myArray.length; i += 1) {
const arrayItem = myArray[i]
if (arrayItem > 1) {
break
}
console.log(arrayItem, i, myArray)
}
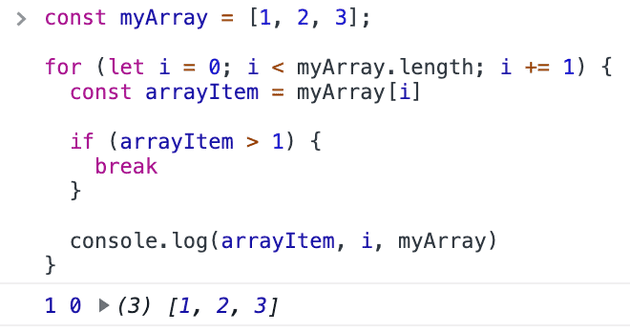
As you can see in the above example, with very little work we can get the for loop to do exactly what the forEach loop does for us except from now we can use the break statement to break out of additional iterations.
Using a while loop as an alternative to a forEach loop to break
A while loop is essentially the same principle as the for loop except that you don’t define the index in the while loop scope.
Here is an example of how you can use the while loop as an alternative to the forEach loop to break with:
const myArray = [1, 2, 3];
let index = 0;
while (index < myArray.length) {
const arrayItem = myArray[index]
if (arrayItem > 1) {
break
}
console.log(arrayItem, index, myArray)
index += 1
}
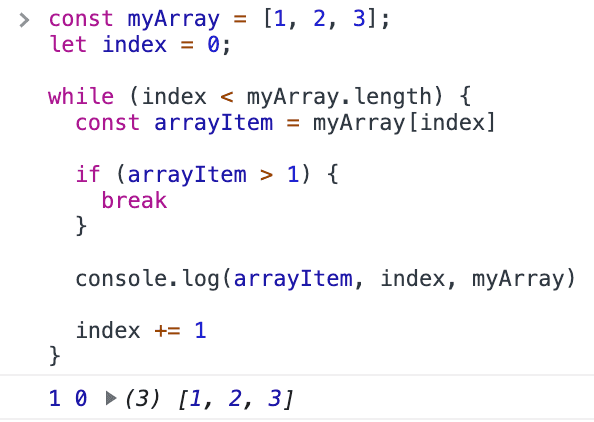
Using a Array.prototype.some as an alternative to a forEach loop to break
The last alternative that you can use for a forEach loop in order to perform a kind of break is the Array.prototype.some method.
You still won’t be able to call the break statement from within it, but instead you are able to return a boolean from your callback which the some loop will use as an indicator to stop iterating over array items.
The original purpose of the Array.prototype.some method is to know if an item exists within an array, if it does the method will return true, if not then it will return false.
We can make use of this to create a break-like functionality by returning true in our callback when we want to emulate a break statement.
Here is an example of how to use the some method to break a loop:
[1, 2, 3].some(a => {
console.log(a)
return true
})
// 1
// true
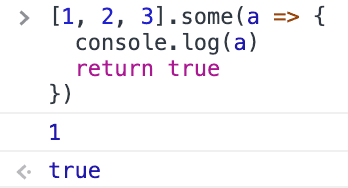
Summary
To summarize this post in a few words, if you need to break out of a JavaScript Array.prototype.forEach then you should not be using a JavaScript Array.prototype.forEach and instead you should be using either a for loop or the Array.prototype.some method.
There we have how to break a JavaScript foreach loop, if you want more like this be sure to check out some of my other posts!