So you have an array of objects and data, and now you want to render it into your react component with JSX?
This post will cover everything you need to know about how to render an array of objects with Array.map() in React that contains any data.
We will be using the Array.prototype.map() function in this guide (We will call it Array.map()
for short in this post) to be able to render our array of objects into our JSX.
If you want to learn more about what the prototype
property is and what it does, I recommend the following post: Inheritance and the prototype chain.
How to render an array of objects with Array.map in React
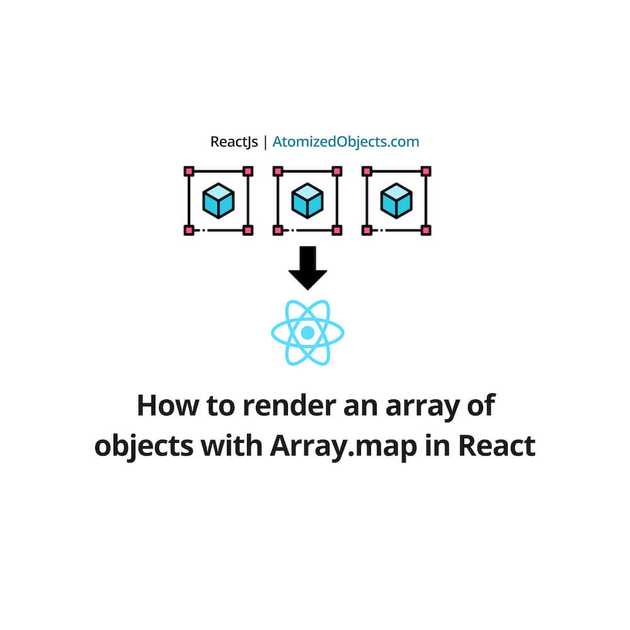
To render an array of objects in react with JSX we need to use Array.map() to transform the object into something react can make use of because you cannot directly render an object into React. Instead, by using Array.map() to convert each object in the array into something else, like a string or a component we can then render it.
const arrayOfObjects = [
{ coffee: "Americano", size: "Medium" },
{ coffee: "Espresso", size: "Single" },
];
export default function MyReactComponent() {
return (
<>
{arrayOfObjects.map(({ coffee, size }) => (
<p key={coffee}>Coffee type {coffee} in a {size} size.</p>
))}
</>
);
}
Transforming arrays of objects and data with Array.map()
The Array.map() method is key to rendering objects and data in react and it is a prototype function on all arrays in JavaScript. This means you can call it on any array with the following syntax: [].map(callback)
.
Array.map is a way to iterate over an array like you would do by using a for loop or Array.forEach
, the difference is that it will create and return a new array after it has finished running.
This syntax is on any array in JavaScript. When you call .map()
you are going to need to provide a callback function for the method to be able to use.
The callback function will be provided with three arguments when it is called by Array.map.
These arguments will be the array item, the index of the array item, and the whole array.
[1, 2, 3].map((arrayItem, arrayItemIndex, wholeArray) => {
arrayItem; // 1
arrayItemIndex; // 0
wholeArray; // [1, 2, 3]
});
For the most part you will only ever need the first two arguments, the array item and the array index.
When we call the Array.map() function in JavaScript it will transform the array into whatever we return from the callback function we pass into it.
This means whatever we return from our callback function will become the new value for that item/index in the array.
So using Array.map in JavaScript will always create a new array.
For example, if we have a small array of numbers 1, and 2 we can use the Array.map() function to convert these numbers into an array 10, and 20 by multiplying each number by 10 and returning it.
Here is how this would look:
[1, 2]; // [1, 2]
[1, 2].map(n => n * 10); // [10, 20]
[1, 2].map(n => `Hello World ${n}`); // [‘Hello World 1', ‘Hello World 2']
Rendering an array of objects in react
Great, so how can we use Array.map() to render an array of objects in react?
Now we understand how to transform a basic array with Array.map, and we know that we cannot render an object into JSX alone, all we need to do next is to apply this to our data.
Firstly let’s create an array of objects and data for use to render:
const arrayOfObjects = [
{ coffee: "Americano", size: "Medium" },
{ coffee: "Espresso", size: "Single" },
];
Now we have some data, we are going to need to transform it into something React can use to render.
To start with we are going to use it to create a list of data to be added into a paragraph element as a string.
Using the Array.map function again, let’s see how our objects with data will now look as React friendly strings.
arrayOfObjects.map(
({ coffee, size }) => `Coffee type ${coffee} in a ${size} size.`
);
Now that will convert our array of objects into an array of strings that we can now use to be able to be rendered into a react component.
Let’s add it into a component now:
const arrayOfObjects = [
{ coffee: "Americano", size: "Medium" },
{ coffee: "Espresso", size: "Single" },
];
export default function MyReactComponent() {
return (
<p>
{arrayOfObjects.map(
({ coffee, size }) => `Coffee type ${coffee} in a ${size} size.`
).join(' ')}
</p>
);
}
Now instead of having this in a single paragraph element we might want these in separate paragraphs rather than all in one, so to do that we are going to need to use a JSX element to create an array of components.
We can return the JSX element from the Array.map in order to create an array of components.
This would then look like the following:
arrayOfObjects.map(({ coffee, size }) => (
<p>Coffee type {coffee} in a {size} size.</p>
));
Now when we use this, it will return an array of components into the JSX which React can then use to render each paragraph into our react component.
You may have noticed that we have had to wrap our array in curly braces, why is it we need to do this?
By adding curly braces into our JSX we can run JavaScript and return the result from the curly braces into the JSX.
For example if you have a string const helloWorld = 'Hello world'
you can render this in JSX by wrapping it in a set of curly braces like so: <p>{helloWorld}</p>
.
Because the variable will resolve as a string with the value of Hello World
, that is what the JSX will render.
The same goes for functions, and all other types of JavaScript.
You can even use the curly braces to run console.log
.
At this point we are missing one key factor in this example.
We need to add in the key
prop for each item in the array.
What is the key prop in react when rendering arrays of data
The key prop is a unique identifier for each item in the array, react uses the key prop to be able to identify and update each item between renders without causing bugs.
If we don’t include it we will get an error message that looks a little like this:

Warning: Each child in a list should have a unique "key" prop.
Check the render method of `MyReactComponent`. See https://reactjs.org/link/warning-keys for more information.
Whenever we render an array of data into a react component it is important to always provide a key so react can track the items between renders.
The best key is going to something unique for each item in your array, so an id, or a name, or a combination of the data in the object.
All we need to do from that point is to pass it in as a prop like so:
arrayOfObjects.map(({ coffee, size }) => (
<p key={coffee}>Coffee type {coffee} in a {size} size.</p>
));
And here is the component making use of this:
const arrayOfObjects = [
{ coffee: "Americano", size: "Medium" },
{ coffee: "Espresso", size: "Single" },
];
export default function MyReactComponent() {
return (
<>
{arrayOfObjects.map(({ coffee, size }) => (
<p key={coffee}>Coffee type {coffee} in a {size} size.</p>
))}
</>
);
}
One thing to remember when it comes to keys in react is that they are local to each array, so you don’t need to worry if two arrays share the same keys or data as long as they are unique in their array.
For example the following is valid and works well because they are different arrays:
const arrayOfObjects = [
{ coffee: "Americano", size: "Single" },
{ coffee: "Espresso", size: "Single" },
];
export default function MyReactComponent() {
return (
<>
{arrayOfObjects.map(({ coffee, size }) => (
<p key={coffee}>{coffee}</p>
))}
{arrayOfObjects.map(({ coffee, size }) => (
<p key={coffee}>{size}</p>
))}
</>
);
}
But if we do the same with the key being used more than once in the same array like the following we would get an error:
const arrayOfObjects = [
{ coffee: "Americano", size: "Single" },
{ coffee: "Espresso", size: "Single" },
];
export default function MyReactComponent() {
return (
<>
{arrayOfObjects.map(({ coffee, size }) => (
<p key={size}>{coffee}</p>
))}
</>
);
}

Warning: Encountered two children with the same key, `Single`. Keys should be unique so that components maintain their identity across updates. Non-unique keys may cause children to be duplicated and/or omitted — the behavior is unsupported and could change in a future version.
Lastly, rending arrays of objects in React will be the same between functional components, class components or pure components in React.
This is because rendering an array of objects or data with map in react will be done with JavaScript and JSX meaning React only uses the result, which would be an array of data formatted in a way JSX can accept.
Summary
That covers pretty much everything you need to know to get started with Array.map to be able to render arrays of objects and data in react.