In this post we will be covering how to break a for loop in JavaScript with as little technical jargon as possible so you will have everything you need right here without having to look any further!
To stop or break out of a for loop in JavaScript is really easy to do thanks to the break statement.
This post will look at all the scenarios you might face when trying to stop or break out of a for loop in JavaScript
How to break a for loop in JavaScript
To stop or break a for loop in JavaScript you need to make use of the break
statement.
Here is a small example of how it looks when using the break statement to stop or break a for loop in JavaScript:
for (let i = 0; i < 10; i += 1) {
if (i === 5) {
break
}
console.log(i) // 0, 1, 2, 3, 4
}
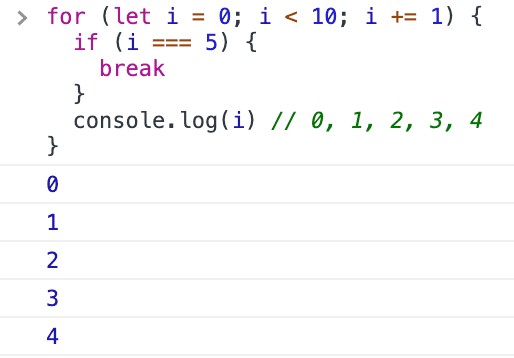
In the above example the for loop will now stop and break when the iterator variable has reached 5 because of the condition in the if statement and then the break statement within it.
It is really that easy and simple to break a for loop in JavaScript.
The same also applies to a while loop and a for of loop in JavaScript.
However it is important to remember that the break statement will not work in any kind of object method such as Array.prototype.forEach or Array.prototype.map.
You might also be wondering where the continue statement comes into play if the break statement is what we use to stop or break a for loop.
The continue statement is used to skip a single iteration in a for loop (or while or for of loop).
This means that whenever we use the continue statement in a for loop in JavaScript we are saying that we don’t want to run any more of the code in the loop.
You can think of the continue statement a little like a return statement in a function.
Once the return statement is triggered, then the function will return any values provided by the return statement and then cancel the rest of the function.
The continue statement does a similar thing with an iteration except you won’t be able to return a value with it.
How to break a nested loop in JavaScript
To break a nested loop in JavaScript can be done in exactly the same way as breaking out of a normal for loop like we have shown above.
The break statement will affect the nearest loop and not any parent loops so this means that if you want to stop or break a nested loop in JavaScript you can use the break statement safely.
for (let i = 0; i < 10; i += 1) {
for (let j = 0; j < 10; j += 1) {
if (j === 5) break
console.log(j) // 0, 1, 2, 3, 4
}
console.log(i) // 0, 1, 2, 3, 4, 5, 6, 7, 8, 9
}
If you do want to break out of both then you will need to create another condition to break out of the parent loop as well.
For example here is how you could break out of both:
let valueFound = false
for (let i = 0; i < 10; i += 1) {
for (let j = 0; j < 10; j += 1) {
if (j === 5) {
valueFound = true
break
}
}
if (valueFound) {
break
}
}
Summary
There we have how to break a for loop in JavaScript, if you want more like this be sure to check out some of my other posts!