This post will cover how to redirect in ReactJs when using react-router and or vanilla js, the possible methods you can use as well as the best practices for each situation.
There are currently many different components, hooks, and methods in which you can redirect using JavaScript and React so this post will add some clarity of how, and why to use them, and for which situations.
We will also be covering external vs internal urls and absolute vs relative and how to handle them all when it comes to redirecting.
I would recommend having a read through my post about how to redirect in JavaScript as well because this will help you understand how not only everything works behind the scenes in React but also it will help understand how you navigate within React as well.
How to redirect in ReactJs
Just before we get stuck into the details of how to redirect in ReactJs, here is a quick cheat sheet to redirecting in React Js / JavaScript (We will go into detail about each later on):
JS - redirect:
window.location.replace("https://google.com/");
JS - user event navigation:
window.location.href = "https://google.com/";
Learn more about the JS implementation here.
React.Js hooks - react-router-dom v6:
const navigate = useNavigation();
navigate("/redirect-example", { replace: true });
React.Js hooks - react-router-dom v5:
const history = useHistory();
history.push("/redirect-example");
React.Js JSX - react-router-dom v5:
import { Redirect } from 'react-router-dom'
…
<Redirect to='/redirect-example' />
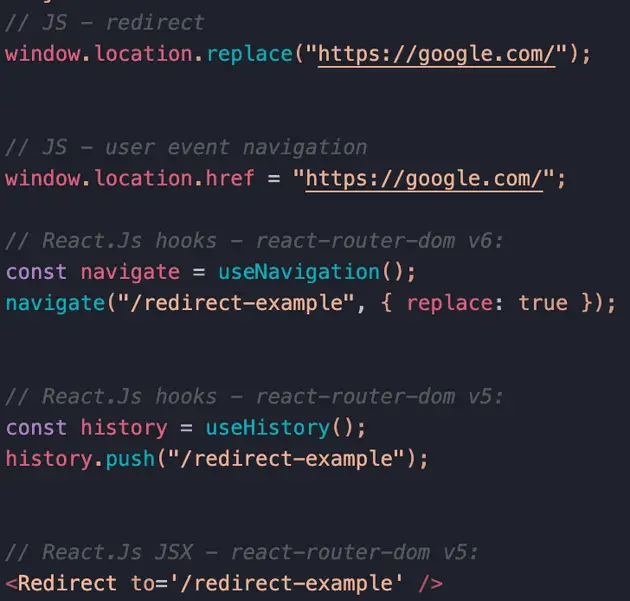
In React there are many libraries that you can use to handle client side routing & navigation and redirection will be a part of those libraries, however the principle of the redirects will be very similar for each.
The principle of a client side redirect will be to push or replace a new route/url to the history of the window in order to change the page.
In vanilla JavaScript you would redirect using window.location.replace, or window.location.href depending on the scenario.
You would use the window.location.replace to perform a redirect which replaces the item in history to prevent loops, and you would use the window.location.href to add onto the history based on a user action.
When it comes to libraries used in ReactJs it will be a similar flow, you will just need to find the specific component/method to use for the given library, such as history.push
.
We will shortly look at the differences between external vs internal urls as well as absolute and relative routes.
But first let’s dive into how to specifically redirect using some popular React libraries such as react-router.
How to redirect in ReactJs with react-router/react-router-dom v6
Firstly we will take a look into how we can redirect in react using the v6 version of react-router and react-router-dom.
It is fairly straightforward and makes use of a hook called useNavigate
which we can just pass our url into with some optional parameters and then it will programmatically navigate/redirect to the new route/url.
Here is an example of the useNavigate
hook in use:
import React from "react";
import { useNavigate } from "react-router-dom";
export default function HookRedirectExample() {
const navigate = useNavigate();
return (
<button
type="button"
onClick={() => navigate("/redirect-example/", { replace: true })}
>
Redirect
</button>
);
}
As you can see in the above example it is fairly straightforward to use, all we need to do is initialize the hook in the component and then call navigate with our url.
I have included the parameter replace: true
which will replace the history entry rather than add to it which is ideal for automatic redirection where you don’t want the user to be able to return to that page directly.
How to redirect in ReactJs with hooks & react-router/react-router-dom v5
Next we will look at the v5 version of the react-router library which is still widely used and we will start by using react hooks, more specifically the useHistory
hook. Making use of the react hook implementation of the history context with useHistory makes it much cleaner and easier to use than in comparison to the JSX option.
All we need to do is import the useHistory
hook from react-router-dom
and then initialise it in a component like so:
import React from "react";
import { useHistory } from "react-router-dom";
export default function HookRedirectExample() {
const history = useHistory();
return null;
}
Once we have a basic setup complete for our component, we then just need to push a new url to the history using history.push("URL_HERE")
whenever a user performs a certain action, in our example we will use a button click.
import React from "react";
import { useHistory } from "react-router-dom";
export default function HookRedirectExample() {
const history = useHistory();
return (
<button type="button" onClick={() => history.push("/redirect-example/")}>
Redirect
</button>
);
}
Now when the user clicks on the button we will push a new relative URL into the history which will cause the user to be redirected to the desired page.
How to redirect in ReactJs with JSX & react-router/react-router-dom v5
Next we will look at how to essentially do the above with a mixture of conditional rendering and the Redirect
component from react-router-dom
.
The principle of the redirect using theRedirect
component is similar to the react hook version although we need to render the Redirect
component instead of calling a function.
This makes it a little less intuitive in comparison to the hook version where you can just call a function as well as slightly different use cases, but it is still a valid way of doing it.
Let’s take our previous example but this time introduce some props to show the real benefit of using the Redirect component to redirect in ReactJS.
import React from "react";
import { Redirect } from "react-router-dom";
export default function HomePage({ authenticated, name }) {
if (!authenticated) {
return <Redirect to="/login" />;
}
return <h1>Welcome {name}</h1>;
}
As you can see in the above example, we are checking to see if the user is authenticated, if not we are rendering the redirect component, and if they are logged in, we are rendering the home page component which displays a welcome message.
If the user is not authenticated the Redirect component will be rendered which will then trigger the page to redirect to the login page.
In summary of the Redirect component it is a nice way to redirect based off of side effects rather than actions.
It is possible to create the same functionality with the hook method as well with the use of useEffect and the dependency array like so:
import React, { useEffect } from "react";
import { useHistory } from "react-router-dom";
export default function HomePage({ authenticated, name }) {
const history = useHistory();
useEffect(() => {
if (!authenticated) {
history.push("/login");
}
}, [authenticated]);
return <h1>Welcome {name}</h1>;
}
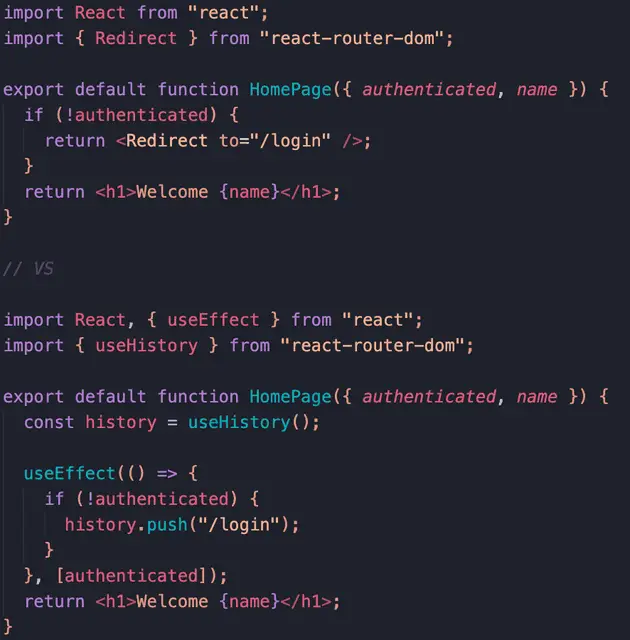
How to redirect in ReactJs with JavaScript
The last redirection method in React is using vanilla JavaScript. React is a JavaScript based library, which means we can run plain JavaScript as well if we need to.
If you are already making use of a routing library, then it would not be recommended to use vanilla JavaScript to navigate or perform redirections because it could interfere with the routing library.
Instead the redirection from vanilla JavaScript is best used with React when you only need very basic navigation where an anchor element can’t do it.
The reason why you might opt for a vanilla JavaScript approach for redirection in React is simple because you might not need to add a whole new library to your application if you have a simple use case, and therefore might make some performance savings by reducing the overall application size.
Here is a quick example of how you can use JavaScript to navigate from a React application:
import React from "react";
import { sendAnalyticsEvent } from "./example-anaylytics.js";
const handleRedirect = () => {
sendAnalyticsEvent("redirect");
window.location.href = "http://google.com/";
};
export default function JsRedirectExample() {
return (
<button type="button" onClick={handleRedirect}>
Redirect
</button>
);
}
Or for an automatic redirect:
import React from "react";
import { sendAnalyticsEvent } from "./example-anaylytics.js";
export default function JsRedirectExample() {
sendAnalyticsEvent("redirect");
window.location.replace("http://google.com/");
return null;
}
Internal urls vs external urls for redirecting in react
The difference between internal and external urls/routes is simple enough where internal urls will navigate within the current website, and external urls/routes will navigate the user to a different website.
The reason why it is important to understand the difference is because handling the different types of url can be very different.
For simplicity let’s assume that we are always talking about urls/routes that will replace the current page (not open in a new tab).
If you are navigating to an external route from your page, you might not need to worry about running it through your library because in most cases the state of the application will be lost after you navigate away from the page in which case using either the React based library or a vanilla JS implementation won’t matter (This may vary between library, so make sure you read the docs!).
If you have an internal route/url though that is managed and handled by a react library you will always want to make use of the libraries implementation or redirection/navigating (even with JSX elements) so that the library can track, manage and render each page whilst maintaining the history.
Absolute urls vs relative urls for redirecting in react
Once again, absolute vs relative urls have a simple difference and it works in the same way as it does when referencing files in a directory.
A relative path is based on where you currently are, but an absolute path does not matter where you currently are and will always take you to the same place.
Generally speaking when it comes to client side applications, a relative path will be relative from the root of the URL/website.
For example, if you are on https://atomizedobjects.com/blog/react/how-to-redirect-in-reactjs/
and you navigate to a relative path of /blog/react
it will take you to https://atomizedobjects.com/blog/react/
.
However if you wanted to do the same thing but as an absolute path you would just need to add the root as well so you would enter https://atomizedobjects.com/blog/react/
instead of /blog/react
.
Summary
There we have how to redirect in ReactJs, if you want more like this be sure to check out some of my other posts!