In this post we will be covering how to redirect in JavaScript with as little technical jargon as possible so you will have everything you need right here without having to look any further!
In this post we are going to look at some of the methods you can use to redirect a page on the client side using JavaScript, or in short, how to redirect in JavaScript.
There are several methods in how you can go about creating a JavaScript redirect, and they are all simple and easy to do, but they have subtle differences between them that are important to learn about and understand before creating a redirect and in this post we will be focussing on window.location.replace and window.location.href.
Just as a quick note, it is generally better to redirect on the BE/server side wherever possible.
But with that said it is not always possible to redirect on the server side which is where this post comes into play with vanilla JavaScript.
How to redirect in JavaScript
In as few words as possible, to redirect in JavaScript (client side redirect) you will want to use window.location.replace.
Here is a quick example of how you can use window.location.replace to redirect in JavaScript:
// relative url / internal link
window.location.replace("/how-to-redirect-in-javascript");
// absolute/external link
window.location.replace("https://google.com/");
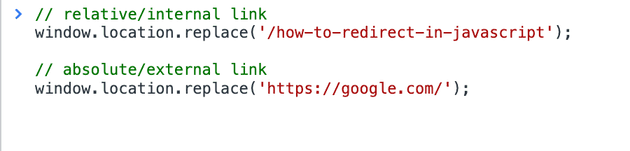
Redirect with window.location.replace in JavaScript
When you make use of window.location.replace you are calling the replace()
method on the Location
interface that is globally accessible in client side/browser JavaScript applications.
You may have seen there are many other methods that seem to do very similar things as the replace method, such as the window.location.assign method, so why do we want to use replace specifically?
Whilst you would be able to make use of window.location.assign to change the current page, it does it in a slightly different way.
When you use the replace method it will actually replace the link/url in the windows history which means that it will be as if the page you are redirecting from did not exist if the user chooses to go back.
When you use window.location.assign, you just add a new entry to the history so that if the user goes back then they will go back to the page you are trying to redirect away from which is not ideal because the user will get suck in a loop where they click the back button, and they get taken back to the page that then redirects them to the page they are trying to go back from.
Here is a flow to show an example of this to help explain it, first with the assign method:
-
User starts at the homepage, ”/“.
-
User navigates to “page-1”.
-
“page-1” uses a client-side redirect to “page-2” implemented using the assign method
window.location.assign('page-2')
. -
User gets navigated/redirected to “page-2”.
-
User clicks back.
-
User gets taken back to “page-1”.
-
User gets navigated/redirected to “page-2” again.
-
Steps 4-6 get repeated over and over.
-
If instead we will look at the same flow but using window.location.replace:
-
User starts at the homepage, ”/“.
-
User navigates to “page-1”.
-
“page-1” uses a client-side redirect to “page-2” implemented using the replace method
window.location.replace('page-2')
. -
User gets navigated/redirected to “page-2”.
-
User clicks back.
-
User gets taken back to ”/” (the homepage).
As you can see the flow that uses the window.location.replace creates a much better user experience because they are able to go back to the page they were on before the redirect however with assign then get stuck being constantly redirected.
That is why for redirection cases it is important to use the window.location.replace method for JavaScript redirection.
Redirect with window.location.href in JavaScript
Another method for how to redirect in JavaScript is to make use of window.location.href. Window.location.href is not actually a method, it is a property that you can manually reassign to change the page that you are on.
When reassigning the window.location.href property, it is essentially doing the same thing as the assign method where you will successfully change the page, however it will be added to the window history which will cause the looping issue we described above.
The reason why it is being included as a method to redirect a page is on a user event such as a mouse click.
If you have an action on your page that requires a mouse click that can’t be done with a HTML anchor element then you might want to use window.location.href to emulate a mouse click because in this situation the user would want to go back to that page because it isn’t an automatic redirect.
Here is an example of how to use window.location.href in JavaScript:
document.getElementById("submit-rating").addEventListener("click", event => {
submitRating(event);
window.location.href = "/thanks-for-rating";
});

Window.location.href vs window.location.replace
To answer what the difference is between window.location.href vs window.location.replace and when you want to use each is a fairly straightforward set of rules.
You should use window.location.replace if you need to create a client side redirect that will always redirect automatically so that it functions properly with the history of a window (prevents back/redirect loop).
You should use window.location.href when you need to redirect based on user events such as a mouse click so that the redirect won’t automatically happen. The reason being is because the user would expect the previous page to be the last page they have seen/interacted with.
Summary
There we have how to redirect in JavaScript, if you want more like this be sure to check out some of my other posts!