In this post we will be covering how to make a loop wait in JavaScript with as little technical jargon as possible so you will have everything you need right here without having to look any further!
It is pretty common in JavaScript to find yourself with a loop that you need to slow down, delay or make it wait for a while.
A common use for needing to make a loop wait in JavaScript would be when polling an API or performing a regular check in an application but to do so without spamming anything too much.
There are many other uses for making a loop wait in JavaScript as well, so let’s take a look at how we can.
How to make a loop wait in JavaScript
To make a loop wait in JavaScript you need to wait for a timed promise to resolve in each iteration of your loop.
This means we need to look at firstly creating a delay in order to slow down the loop and make it wait, and we also are going to need to look at the loop itself to make sure that everything is working as it should.
Let’s take a look at how we can create an asynchronous delay function to slow down and make the loop wait.
const delay = async (ms = 1000) =>
new Promise(resolve => setTimeout(resolve, ms))
This sleep/delay function we have created accepts the amount of time we want to wait for in milliseconds (ms) and then returns a new promise that will resolve after the amount of time we have provided it.
We prevent it resolving right away by using the setTimout
method which will only call the function we provide it after the time we have provided it has completed.
You can think of the setTimeout method like a timer for our function.
The next thing we need to look at to make a loop wait in JavaScript is the loop itself.
For this solution we will make use of the standard JavaScript for loop because it works easily with asynchronous code like our delay function, but any kind of for loop or while loop will also work.
Let’s take a look at how we can use the for loop with our delay function:
async function makeALoopWait() {
const exampleArray = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
for (let i = 0; i < exampleArray.length; i += 1) {
console.log(i)
await delay(1000)
}
}
makeALoopWait()
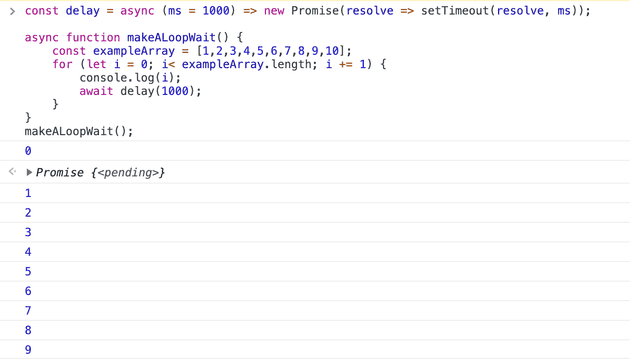
You will notice that in this snippet I have wrapped the for loop in an asynchronous function, which enables us to run asynchronous code, like await
which we need so that we can tell our JavaScript code to wait for the delay/sleep function to finish before moving on to the next iteration.
The for loop itself is pretty standard and nothing special, the only part that is different here is that we are calling await delay(1000)
from within the for loop block which is waiting for the delay function that we created to complete which will take 1 second because we are passing in 1000 milliseconds as the time it needs to wait.
So in this example this loop will now print out the array item every second to the console.
And to see all the completed code for how to make a loop wait in JavaScript:
const delay = async (ms = 1000) =>
new Promise(resolve => setTimeout(resolve, ms))
async function makeALoopWait() {
const exampleArray = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
for (let i = 0; i < exampleArray.length; i += 1) {
// Your code goes after this line!
console.log(i)
// Your code must finish before this line!
await delay(5000)
}
}
makeALoopWait()
To change this so that you can use this with your code, just insert your code between the comments.
It is worth mentioning that if you want to run your function at regular intervals for the lifetime of the application, it might be easier to look into using setInterval to run your function at regular intervals instead of a delayed for loop.
You can use setInterval in the same way you would the setTimeout but instead of only running the function once after the timer has completed, it will reset the timer and run your function again at each interval.
To make a for loop wait for a callback
It is pretty much the same principle as above to make a for loop wait for a callback, but instead of needing to create a delay or sleep function, all we need to do is wait for the callback.
async function makeALoopWait() {
const exampleArray = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
for (let i = 0; i < exampleArray.length; i += 1) {
await callback()
}
}
makeALoopWait()
Why you can’t make a forEach loop wait in JavaScript
You might be wondering why I have chosen to use a for loop instead of using the forEach method or the map method.
The reason is because the methods forEach and map are not asynchronous which means they will not be able to wait for an asynchronous function to complete before moving onto the next one so it will end up just ignoring the asynchronous delay function that we created instead of waiting.
Summary
There we have how to make a loop wait in JavaScript, if you want more like this be sure to check out some of my other posts!