In this post we will be covering findIndex vs indexOf - JavaScript with as little technical jargon as possible so you will have everything you need right here without having to look any further!
As you know there are two helpful methods for finding an index in a JavaScript array, but which one should you use?
In this post about findIndex vs indexOf in JavaScript we are going to look at the differences between the Array.prototype.findIndex method and the Array.prototype.indexOf method.
Before we get stuck into this article I have two posts, how to get the index of an item in an array in JavaScript, and how to get the index of an object in an array in JavaScript, that go into detail about how to use both of these methods and I would highly recommend having a read of them after this post.
Now, let’s get stuck in and see what the differences are between findIndex vs indexOf.
What is the difference between findIndex vs indexOf in JavaScript?
The main difference between findIndex vs indexOf in JavaScript is that findIndex accepts a callback as an argument, and indexOf accepts a value as an argument.
What this means is that indexOf will only ever look for a value in an array, whereas findIndex will let you decide how you find the index.
Here is a visual example of the differences between Array.prototype.findIndex method vs the Array.prototype.indexOf method:
const exampleArray = ["a", "b", "c"]
exampleArray.indexOf("b") // 1
exampleArray.findIndex(arrayItem => arrayItem === "b") // 1
exampleArray.indexOf("d") // -1
exampleArray.findIndex(arrayItem => arrayItem === "d") // -1
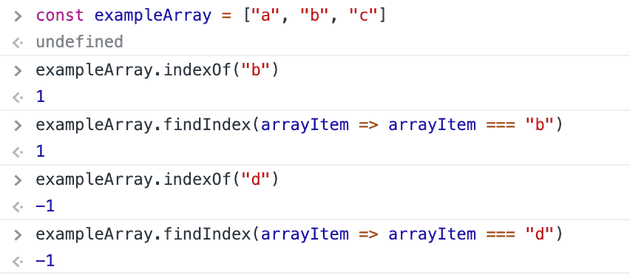
As you can see both are very similar and give you the same result when looking for the same value.
The callback function we provide to findIndex always needs to return a boolean value. If the callback function returns true, the findIndex method will consider the array item to match what we are looking for and it will return that index.
If the callback returns false it will move onto the next array item.
Both methods will return -1 if they cannot find the correct index before the end of the array.
When using indexOf it will look through each array item and return the first index it finds that matches the value we provided to it, if it does not find anything it will return -1.
When using findIndex it will pass each array item into the callback we provide it, when our callback returns true it will know that it has found the index which it will return to us. If it does not find an index, or if the result from every callback is false then it will return -1 just like indexOf because it has not been able to find the index.
You might now be wondering what the need is to have the callback function in this example and why shouldn’t you just use the indexOf method?
The reason is because in this example we are using very simple values in the array, which is what Array.prototype.indexOf does really well, but if we needed to look for the index of something more complex like an object, that is where things get a little trickier.
If you are looking for an object, unless you can provide referential equality, you won’t be able to use indexOf because two identical objects are not equal.
I go into more detail about this in my post about how to get the index of an object in an array in JavaScript.
But to cover it briefly here, the only time an object comparison will return true is if you are comparing the same object.
Here is an example of this:
{} === {} // false
const myObj = {}
myObj === myObj // true
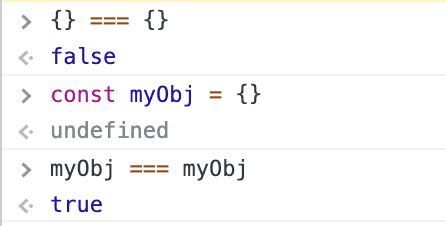
Even though the objects are identical, because they do not point to the same reference the comparison fails, but when they do point to the same reference the comparison passes.
With this in mind here is an example of how finding the index of an object in an array in JavaScript works when looking at findIndex vs indexOf:
const exampleObj = { laptops: 5 }
const exampleArray = [{ laptops: 10 }, exampleObj, { laptops: 14 }]
exampleArray.indexOf({ laptops: 5 }) // -1
exampleArray.indexOf({ laptops: 10 }) // -1
exampleArray.findIndex(arrayItem => arrayItem.laptops === 5) // 1
exampleArray.indexOf(exampleObj) // 1
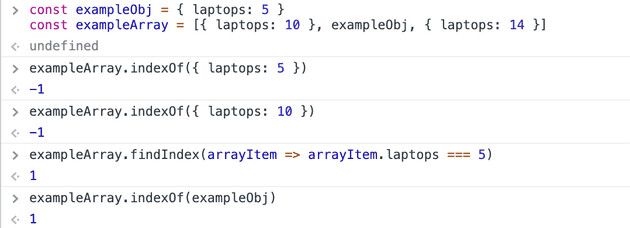
As you can see with indexOf even though we are providing an identical object, it cannot find it in the array.
Using findIndex allows us to check the properties, keys and values within each object in an array and it can therefore find the correct item in the array and return the index two us.
And lastly, because we are passing the reference into the last indexOf method it can find the correct item in the array because of referential equality.
Should you use findIndex vs indexOf in JavaScript?
If you are searching for a simple value like a number or a string, for the most part you will probably want to use Array.prototype.indexOf because it does exactly what you need and it is easy to use and understand.
If you are searching for a more complex value like an object then you will most likely want to use Array.prototype.findIndex so that you can find the correct index by searching with the keys, values and properties of each object.
There is one small edgecase where you can use indexOf to search for an object as long as you have referential equality, but this could cause confusion and in most cases won’t be particularly useful, so even if you do it would still probably be worthwhile going with Array.prototype.findIndex.
Performance of findIndex vs indexOf in JavaScript
In general the performance between findIndex vs indexOf is going to be completely negligible, and therefore you should not worry about it.
With this being said, it is dependent on the array you use for both, and the callback function you provide the findIndex method.
If you are doing many complex computations in the callback you provide then it will be worse in performance than indexOf, but at that point it would not be a fair comparison because indexOf would not be able to be used anyway.
If we assume that the callback you provide is a simple assertion or condition that returns a boolean, then both of these methods will run in the same amount of space time complexity (big O notation) which is O(n)
in the worst case scenario.
If do decide you want to look into the performance differences in more detail, I suggest you take a look at indexOf and findIndex here to see how both of these methods work under the hood.
But remember, both have the same space time complexity and will offer very little performance improvements between the two so it is therefore almost certainly not worth optimizing the performance between these two methods.
If you are finding that you are having a performance issue when using the findIndex method, it will most likely be because the callback function you are providing could be doing too much work, or the array size is too large.
Summary
There we have findIndex vs indexOf - JavaScript, if you want more like this be sure to check out some of my other posts!