In this post we will be covering how to get the index of an item in an array in JavaScript with as little technical jargon as possible so you will have everything you need right here without having to look any further!
Finding the index of an item in an array in JavaScript can be pretty useful at times and is something that you will likely need to do many times for one reason or another.
We can do this by looking at the Array.prototype.indexOf method or the Array.prototype.findIndex method.
Before we get started, this post will mostly be looking at the indexOf method because it does exactly what we need, if instead you want a more detailed explanation of the findIndex method, or how to get the index of an object in an array in JavaScript I have written an in-depth article explaining these topics here.
Let’s get started with how to get the index of an item in an array in JavaScript.
How to get the index of an item in an array in JavaScript
The easiest way to get the index of an item in an array in JavaScript is by making use of the Array.prototype.indexOf method. By calling indexOf on any JavaScript array you can pass in an argument for the item you are looking for and it will return the index of it, or -1
if it cannot find it.
Here is an example of how you can use indexOf to get the index of an item in an array in JavaScript:
const arrayExample = ["a", "b", "c", "d"]
arrayExample.indexOf("b") // 1
arrayExample.indexOf("e") // -1
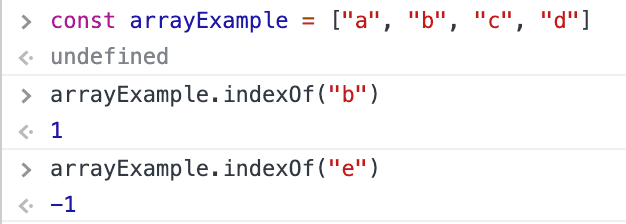
As you can see in this example all we are doing is calling the indexOf method on our array and passing in the item we are looking for.
If it finds the item, then it returns the index, if it cannot find the item it will return -1 so that we know it has not found the item.
This works well for many kinds of arrays, but if we have a slightly more complex array such as an array of objects, then instead we can use the Array.prototype.findIndex method.
Here is an example of how to use the Array.prototype.findIndex method to get the index of an item in an array in JavaScript:
const arrayExample = ["a", "b", "c", "d"]
arrayExample.findIndex(arrayItem => arrayItem === "b") // 1
arrayExample.indexOf(arrayItem => arrayItem === "e") // -1
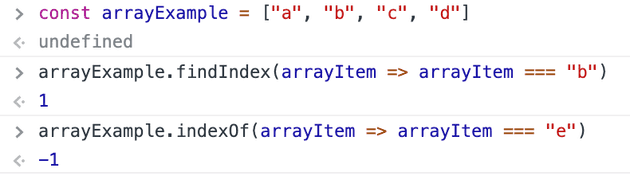
Using this method lets us create our own callback function to check if the item in the array is the correct index.
If we return true in the callback function then the findIndex method will consider that item to be the correct index and will return the index to us.
If we return false in the callback function then the findIndex method will consider that item to not match the item you are looking for and for it to carry on to the next item in the array.
If it can’t find the correct item in the array, then it will return -1
instead, just like in the indexOf method.
For more detail about how you can use Array.prototype.findIndex to get the index of an object in an array in JavaScript, read my post about it here.
But here is a quick example of how you can do so:
const anArray = [{ oranges: 8 }, { oranges: 12 }, { oranges: 6 }]
anArray.findIndex(arrayItem => arrayItem.oranges === 12) // 1

Summary
There we have how to get the index of an item in an array in JavaScript, if you want more like this be sure to check out some of my other posts!