For the most part, you aren’t going to need to use useDebugValue in your React hooks, but sometimes it can be helpful depending on how complex your custom React hook is.
We are going to go over the hook useDebugValue, what it is, how to use it and then when you should use it.
There will be examples of useDebugValue for each part as well!
As with all of my articles, I will be writing in plain english without technical jargon so that everyone, no matter what experience level can understand and then use this hook.
So let’s get started with useDebugValue!
What is useDebugValue in React?
The hook useDebugValue works hand in hand with the react dev tools from Facebook.
It enables you to be able to log information in the dev tool in an easier format than just seeing the values of the hook.
Here is how the react dev console looks:
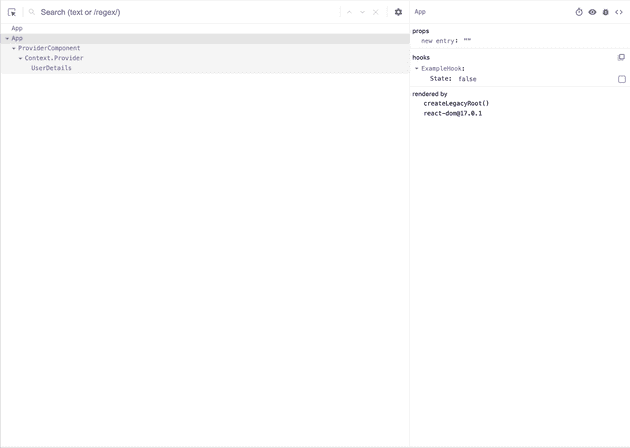
Here is how an example hook might look without the use of the useDebugValue react hook:

And here is how our example hook will look in the react dev console after using useDebugValue:
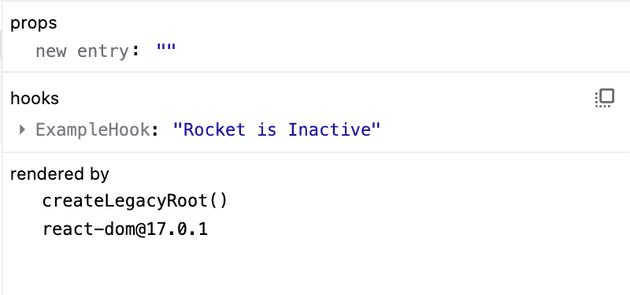
In this example the state is pretty simple to start with (Just a single boolean), but even then, using useDebugValue can add some much needed context to the hook.
As your custom hooks grow in complexity, you may find that if you need to debug them, it will be much simpler to be able to just glance at a string like in the photo above that tells you what state the hook is in.
What about console.log?
Personally, my go to for debugging JavaScript and React code is to use console.log, and I would be lying if I said I would use useDebugValue instead of console.log.
Console.log provides a quick, easy, universal way to debug your code with very little work and without having to import any functions or new code to make it work.
With that being said, there are definitely times that you would want to use useDebugValue over console logging which we will go over a little later on.
How to use useDebugValue in your react hook.
Using the react hook useDebugValue is pretty straightforward, you just need to call the function inside of a custom react hook along with a string that will be used to debug with.
Here is a useDebugValue example:
import React, { useDebugValue, useState } from "react"
export default function useExampleHook() {
const [b, setB] = useState(false)
useDebugValue("Rocket is Inactive")
return [b, setB]
}
export default function App() {
useExampleHook()
return <div />
}
As you can see, this could have a lot of potential if we use it to highlight the current status of the hook.
To do that we just need to change the string conditionally, or we can call the function conditionally.
In the next example you will see it being used to determine the basic status of the custom hook by conditionally choosing which string to display in react dev tools.
import React, { useDebugValue, useState } from "react"
export default function useExampleHook() {
const [b, setB] = useState(false)
useDebugValue(b ? "Rocket is Active" : "Rocket is Inactive")
return [b, setB]
}
export default function App() {
useExampleHook()
return <div />
}
When to use useDebugValue in React?
You should only use this hook in combination with a custom React hook.
Even then, it doesn’t mean you need to add it to each and every custom react hook you make either.
You only really need to add it if you have a complex hook that you may need to re-visit in the future, or if you are making a react hook as part of a library and the hook’s state is not immediately obvious from looking at the values in the hook.
Simply put, use it sparingly because for the most part it won’t be needed.
With that said, it is always a good thing to know how to use it so you can add another tool to your toolbox for the future.
To summarize useDebugValue
In summary, useDebugValue is a hook which will allow you to improve debugging hooks by letting you write a human readable string which will be easier to read and debug than values would be.
I hope you liked this article, please check out some of my others!