In this post we will be covering how to use dangerouslySetInnerHTML in React with as little technical jargon as possible so you will have everything you need right here without having to look any further!
In React, you might come across having a need to set HTML directly that you have as a string.
That is where dangerouslySetInnerHTML comes into play in React components which enables you to be able to set HTML within JSX elements.
In this post we are going to cover what dangerouslySetInnerHTML is in React components, how to use dangerouslySetInnerHTML, using dangerouslySetInnerHTML with script tags, using dangerouslySetInnerHTML without div elements, and more!
What is dangerouslySetInnerHTML in React?
In React, dangerouslySetInnerHTML is a way in which you can directly pass HTML into an element within JSX.
For the most part you can use JSX to create a HTML-like syntax that will be used to render components and elements as HTML but occasionally you might want to render a string of HTML instead which you can’t do directly in JSX.
When you use dangerouslySetInnerHTML it accepts a string of HTML rather than JSX like syntax which makes it easy to pass in any kind of HTML you want even if it is from an external source like an API.
A common use for this could be adding inline scripts by using dangerouslySetInnerHTML with a script tag in the head or body of your application, or by rendering HTML that is returned from an API.
How to use dangerouslySetInnerHTML in React
You can use dangerouslySetInnerHTML on any JSX element, however you can’t use it directly on your React components unless you create a component that can accept it.
This means that you can use dangerouslySetInnerHTML without a div element as well, and instead use it on span elements, script tags, li elements, as well as div elements and much more.
Let’s take a look at an example of how to use dangerouslySetInnerHTML in React with a JSX element:
export function exampleComponent() {
const htmlString = "<p>This is some HTML being set as a paragraph</p>"
return (
<div>
<h1> Hello World </h1>
<div dangerouslySetInnerHTML={{ __html: htmlString }} />
</div>
)
}
The above component will then be rendered as the following HTML:
<div>
<h1>Hello World</h1>
<div>
<p>This is some HTML being set as a paragraph</p>
</div>
</div>
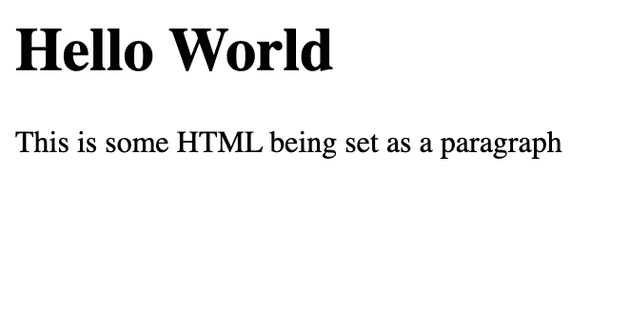
Just to cover all scenarios of using dangerouslySetInnerHTML let’s look at two more examples, one using a script tag and one without the div element.
dangerouslySetInnerHTML script tag example:
export function exampleComponent() {
const inlineScript = '(() => { console.log("Hello World!") })();'
return (
<script
type="text/javascript"
dangerouslySetInnerHTML={{ __html: inlineScript }}
/>
)
}
This will now log “Hello World!” whenever this script tag gets run.

dangerouslySetInnerHTML without div example:
export function exampleComponent() {
const htmlString =
"This is some <strong>HTML</strong> being set as a paragraph"
return (
<div>
<h1> Hello World </h1>
<p dangerouslySetInnerHTML={{ __html: htmlString }} />
</div>
)
}
This will now bold the word “HTML” because we are setting the inner HTML of the p element with our string with the “HTML” word wrapped in a strong element.
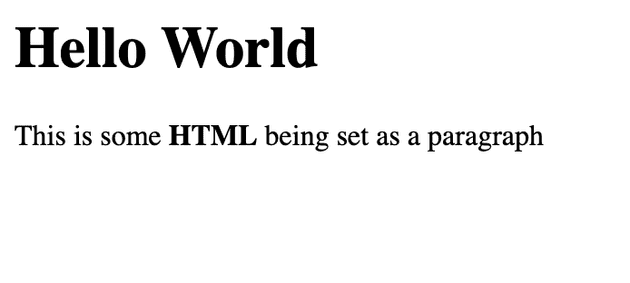
Render HTML without dangerouslySetInnerHTML in React
To quickly go over how you can render HTML in React without using dangerouslySetInnerHTML, it is important to remember what React aims to do and how JSX is used.
When using React any JSX you use will be returned and rendered as HTML and therefore if you want to render HTML without dangerouslySetInnerHTML, you can and should do it within JSX.
Using dangerouslySetInnerHTML should only be used when you have no other option but to use it. If you can use JSX to define and render your HTML elements then you should.
Why there are no dangerouslySetInnerHTML React alternatives.
The problem of looking for alternatives primarily comes from the naming of this prop which is dangerouslySetInnerHTML
.
It sounds worrying because you know you are doing something dangerous because of the name, but actually it isn’t as dangerous as it may feel or sound.
The naming of dangerouslySetInnerHTML was intentional from the React team as a reminder to you to be careful when using it.
The reason why dangerouslySetInnerHTML
is called as such is because one of the primary uses for this prop is to set HTML that you get given from an API and you therefore have no control over.
This means that if you set HTML from another origin they could send you malicious HTML that you will then set and run in your application which could run unknown JavaScript and more which is a problem.
However, if you are confident that this HTML is safe, or if is HTML that you have written yourself then it is not actually dangerous as long as it is valid HTML.
So you can consider it a reminder to make sure you are careful of the source of the HTML when using dangerouslySetInnerHTML.
Aside from that, there is one alternative to using dangerouslySetInnerHTML which is to just set the innerHTML of an element using vanilla JS instead.
Vanilla JavaScript alternative example:
document.getElementsByTagName(‘p’)[0].innerHTML = "This is some <strong>HTML</strong> being set as a paragraph"
Setting the innerHTML using vanilla JavaScript like this however is not ideal in a React application because by setting dangerouslySetInnerHTML you are informing React not to overwrite this HTML in any dyrating or re-rendering steps which is important otherwise you could lose the html.
Summary
There we have how to use dangerouslySetInnerHTML in React, if you want more like this be sure to check out some of my other posts!