In this post we will be covering how to use css variables with React with as little technical jargon as possible so you will have everything you need right here without having to look any further!
Why should you use css variables with React
In React there are many different ways to go about styles and themes, but all of them end up as css and inline styles one way or another.
Personally I am not a fan of using libraries for theming and styling react based applications because it adds an extra layer of complexity when it can usually be avoided.
This is where css variables come into play in React applications, one of the main points for using libraries and inline styles in React applications are because of the ease of using variables in the styles so you don’t need to constantly re-define them.
The alternative to this previously was to use something like scss or less to handle the variables for you, but now with css variables this functionality is built in so we can streamline React applications by removing the extra javascript code, and libraries, and now by removing the build steps for having to convert scss and less into css for the browser.
All in all this means that by using css variables we can avoid any build steps and extra code which will either reduce the build time and make the pipeline cheaper and more efficient to run, or it will reduce the amount of javascript code we are sending to the client which will improve load times and performance.
How to use css variables with React
Css variables are supported in most browsers so in order to use css variables with React all we need to do is write some css.
You can define css variables by using two hyphens, when you define the variable it is important to remember that whatever selector you use will be where the variable scope can exist.
For example, if you define a variable on the body tag then the variable will be accessible to all child and sub child elements of the body so essentially making the variable global.
body {
--primary-color: #000;
}
After you have defined your variables, to use them all you have to do is to reference them as a value using the var
keyword.
You can do this like so:
.someClass {
background-color: var(--primary-color);
}
This principle can be used across the entire react application as long as your css with the variables has been imported into the application, this might be worth importing at the root level.
Once these variables have been set you can use them in any style across the application, which can be inline-styles, css, css modules, scss, less and so on.
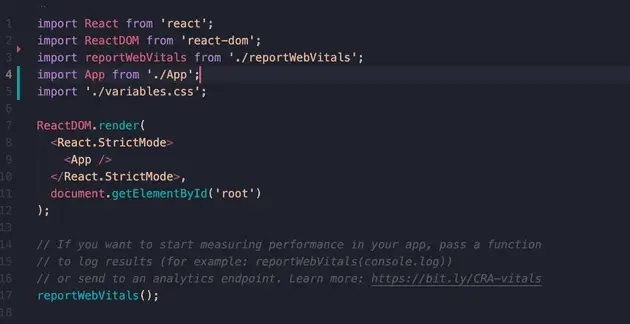
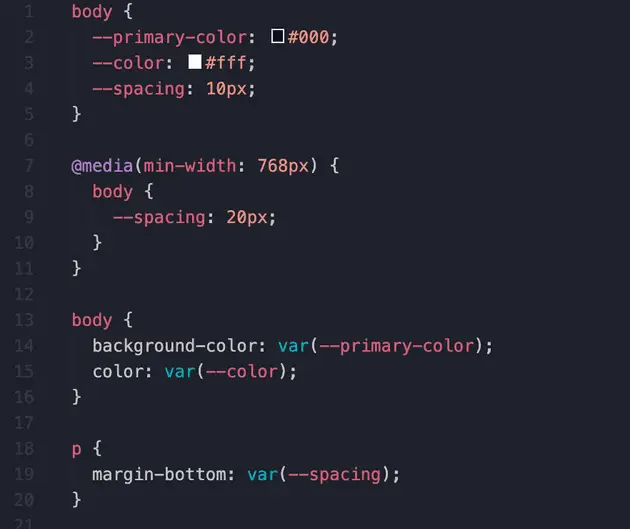
Media Queries with css variables
In most web applications you will need to make use of media queries and it is no different with React applications.
Unfortunately you can’t use css variables to create media query breakpoints, but you can use media query breakpoints to change the css variables so that whenever you need to have something change at different viewports and widths, you can do it at the variable level so you don’t need to worry about it for the various components.
For example let’s take a look at the following css:
body {
--spacing: 10px;
}
@media (min-width: 768px) {
body {
--spacing: 20px;
}
}
In the above example whenever you use the spacing
variable, you no longer need to provide additional media queries in your component to change the spacing depending on the width, instead the variable value will change.
So for smaller mobile devices the spacing variable will equal 10px
, but for anything with a screen width over 768px
the spacing variable will be equal to 20px.
This means you can keep most (possibly even all) of your media queries in a single place and have dynamic variable values which handle the rest, so you no longer need to worry about sharing the breakpoints across your application.
This does mean that you need to consider the design system before starting the build process so you can effectively create components that work in combination.
A simple example for this process would look something like:
- Designs complete
- Build component library with all shared variables and media queries in a single file
- Ensure all components match behaviour in design using css variables.
- Plug and play components however you choose in your application.
How to use css variables inline
One of the benefits of css variables is that it is also possible to use the variables inline which is not something you can do when using scss, less or various other technologies.
To use css variables inline you just need to reference them just like you would do in standard css.
Here is an example of using css variables inline in a react application:
export default function Box({ children }) {
return (
<div style={{ backgroundColor: "var(--primary-color)" }}>{children}</div>
);
}
How to set global css variables for React
Setting global css variables for React is just a case of setting the variables on either the root or the body of the application.
As long as they are defined at the top level all child elements will have access to them.
Theming with css variables in React
One of the main benefits of css variables in React is theming, it has always been a bit of a pain to create themes in React applications because most of the time it involves using a theme provider and react context.
However, now with css variables it is just a case of re-defining variables to change the theme.
An example of this could be that you will have a root file with all of your variables and media queries that define the standard colours, sizes, media queries which would be using the body
as a selector.
Then you can create a theme file alongside this which alters the variables for your given theme, which could change the font family, colorus, sizes and so on.
The difference would be that in the themed file you would use a class on the body to define the variables such as body.theme
.
You would import both of these css files into the root of your application and then using React and JavaScript you can toggle the theme
class on and off to then show a different theme to the user without ever needing to add any form of context.
Here is a basic example of how you can do this in React:
/* variables.css */
html, body, #root {
margin: 0;
padding: 0;
width: 100%;
display: block;
}
#example-app {
--primary-color: #000;
--color: #fff;
--spacing: 10px;
}
@media(min-width: 768px) {
#example-app {
--spacing: 20px;
}
}
#example-app {
display: block;
width: 100%;
background-color: var(--primary-color);
color: var(--color);
padding: var(--spacing);
}
h1 {
margin-top: 0;
}
p, h1, button {
margin-bottom: var(--spacing);
}
/* light-theme.css */
#example-app.light {
--primary-color: #fff;
--color: #000;
}
/* index.js */
import React, { useState } from 'react';
import ReactDOM from 'react-dom';
import './variables.css';
import './light-theme.css'
const App = () => {
const [lightTheme, setLightTheme] = useState(false);
return (
<div id="example-app" className={lightTheme ? "light" : "dark"}>
<h1>Hello World</h1>
<p>This is an example of using themes with css variables</p>
<button onClick={() => setLightTheme(s => !s)}>Toggle Theme</button>
</div>
)
}
ReactDOM.render(
<React.StrictMode>
<App />
</React.StrictMode>,
document.getElementById('root')
);
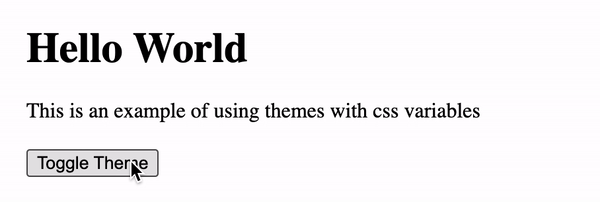
Summary
There we have how to use css variables with React, if you want more like this be sure to check out some of my other posts!