In this post we will be covering how to show and hide components and elements in React with as little technical jargon as possible so you will have everything you need right here without having to look any further!
In frontend development generally one of the things you will find yourself doing fairly often is showing and hiding components and elements.
This is no different with react, you will need to know how to show and hide elements and components as well as the various different ways you can do it.
How to show and hide components and elements in React
To show and hide components and elements in React you will need to either use conditional rendering, css styles or animation libraries.
For the most part conditional rendering will be done by checking if a value passes a condition, just like in an if statement but for react components and JSX.
Once you know how to control the element or component the choice will then be whether you need to conditionally render the component or element with React and JavaScript or if you simply want to hide the element with css.
When you conditionally render a component you are actually adding or removing it from the DOM.
When you show or hide an element with css the element is always there, just not visible to the user.
There can be cases where you could want both hiding and removing and that is when doing various animations.
For the most part, if you can remove additional elements from the DOM it will be worthwhile doing in order to reduce the overall size of your DOM tree which can help to improve the loading time for users.
How to show and hide elements in React
To show and hide elements in React with css there are a number of ways in which you can do it.
However all of them will need a handful of css properties that need to be in place to not only hide the element, but to make sure the users don’t accidentally click on the element or get blocked by the element.
When showing and hiding elements you need to make sure that you will have full browser support as well because css does not always act equally between different browsers.
So let’s take a look at the properties of a properly hidden element.
.example-properties {
display: none;
opacity: 0;
pointer-events: none;
visibility: none;
z-index: -1;
}
Now you won’t necessarily need all of these properties all at once but you will almost certainly need a combination of them depending on your use case.
Let’s now take a look at a few of these use cases.
Display none
If you are using display: none
to hide your element in React then you will probably be better off conditionally rendering the element/component instead because chances are you won’t need it in the DOM tree and you can’t animate or transition a display: none
.
With that said, a tip when using display: none to hide an element is to apply it to hide the element, but to remove it when you need to show the element.
This might sound obvious but you need to make sure you don’t re-define it as display: block
, or display: inline-block
, and so on because it can cause bugs in the UI.
Opacity, pointer-events, and z-index
When you use a combination of opacity, pointer-events and z-index, chances are you are dealing with some kind of modal/lightbox and want to fade it in and out which you cannot do with display: none or removing the element from the DOM.
To create this fading effect you will also need to combine the styles above with a transition
as well.
Here is the css to show/hide an element with a fade effect:
.lightbox {
position: absolute;
display: block;
top: 0;
bottom: 0;
left: 0;
right: 0;
background: rgba(0, 0, 0, 0.5);
transition: 0.3s all;
opacity: 1;
pointer-events: auto;
z-index: 1;
}
.hide-lightbox {
opacity: 0;
pointer-events: none;
z-index: -1;
}
Once you have your css defined it is just a case of adding or removing the hide-lightbox
class with React.
Then once you combine this with the following react example:
import React, { useState } from "react";
import "./base.css";
import "./lightbox.css";
export default function App() {
const [hideLightbox, setHideLightbox] = useState(true);
return (
<>
<button onClick={() => setHideLightbox(false)}>Show Lightbox</button>
<div className={`lightbox ${hideLightbox ? "hide-lightbox" : ""}`} />
</>
);
}
Here is how it looks to have a fading show/hide element in React:
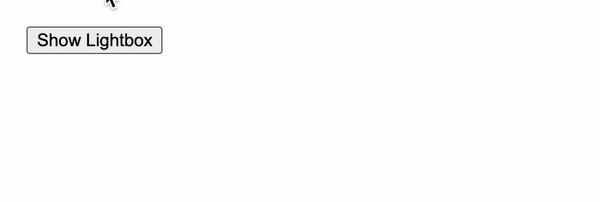
Visibility
The visibility property is slightly similar to using display: none
as in it will determine if the element is visible or not, however unlike display: none
, visibility
won’t completely hide the element from the DOM, it will just make it invisible so that the space where the element is will still be there, it is only the element itself that won’t be visible.
To put it simply, display: none
will hide an element as if it has been removed from the DOM leaving no evidence aside from in the DOM tree.
Whereas visibility: hidden
will make an element invisible but it will leave evidence of its existence because the space created by the element will still be there because it is just invisible.
In a way, you can think of visibility: hidden
as setting the opacity
to 0.
How to show and hide components in React
To show and hide components in React you are going to need to conditionally render them.
Conditionally rendering a component is just a way of saying that depending on an if statement, we might return one component or another.
In most simple cases this is simplified even further because we can do this directly in JSX and instead of returning one of two components we can return the component on a successful condition otherwise we can just return null or undefined for react to ignore it instead, or in other words hiding the react component.
Here is how conditional rendering looks in JSX:
<>
{true ? <p>Hello World</p> : null}
{true && <p>Hello World</p>}
</>
In the above example it will always render the components because our condition is just using true
which is the equivalent of adding true
into an if statement so it will always pass.
The reason why we can’t just show or hide components with css is because a component is not an element.
A react component can contain elements, and you can even create components that show and hide with the use of css.
But for most components, unless specifically built in as functionality, to show or hide them you will need to conditionally render them.
Here is an example of hiding a react component by conditionally rendering it.
// React show/hide example on click
import React, { useState } from "react";
export default function App() {
const [hidden, setHidden] = useState(true);
return (
<div>
<h1> Hello World </h1>
{!hidden ? <p>You can see me!</p> : null}
<button onClick={() => setHidden(s => !s)}>
react show hide component
</button>
</div>
);
}
As you can see, in our JSX we are creating a block of executable JavaScript code that returns either a React component or null.
If the condition passes then we render the component, otherwise we do not, and this is what conditional rendering is.
You can use just about anything for the condition to conditionally render the component, but common conditions that are used are usually, enums, state, props, the existence or absence of a prop, or a combination of all of them.
There are no set rules here and it is an easy and convenient way to structure and render components in your JSX based on the data you have.
There are some exceptions which can be components that have been specifically designed to handle this, or animation based components.
If you do need to animate your components when hiding or showing them, I highly recommend using React Spring.
Display none vs conditional rendering in React
When in doubt, if there are no animations attached to the show/hide part of your application then it is almost always better to conditionally render them to prevent adding extra elements into your DOM tree.
The smaller the tree, the faster your application is likely to run which will benefit the user.
There are various different use cases though, so this could be dependent on what your specific need is, but as a rule of thumb if the goal is to make it seem like the element is not there, and there are no transitions or animations then conditional rendering is probably the best option.
The reason being is because it is not possible to add transitions to display: none
.
Summary
There we have how to show and hide components and elements in React, if you want more like this be sure to check out some of my other posts!