In this post we will be covering what is JSONP and How to use JSONP in JavaScript with as little technical jargon as possible so you will have everything you need right here without having to look any further!
Before we get started, it is worth noting, in most cases you shouldn’t really use or have a need to use JSONP(JSON-P) anymore. So unless you have no control over it, it is best to avoid it.
In practice you won’t find it in use for the most part.
Personally, the only time I have ever needed to use JSONP was part of a technical interview test (which is quite a strange one to see in an interview in my opinion).
Anyway, let’s begin.
What is JSONP (JSON-P)?
The easiest way to think of JSONP is that instead of loading in JSON data, you are loading in a JavaScript resource like you normally would, but it comes along with all the data you need as well.
JSONP is very similar to JSON (JavaScript Object Notation) in pretty much all aspects except from how JSONP is accessible via a callback function rather than parsing the JSON directly.
JSONP stands for JSON with Padding, the “Padding” here being the callback function.
What this means is that you can request data in the form of JSONP which then gets loaded into your page. When it finishes loading in it will call whichever callback function was provided which gives you the ability to then use that data.
To break this down a little more here is what JSONP is and does in a step by step list:
- You import JSONP data via a script tag in your HTML.
- When it loads in from the resource, it will call a callback function of your choice with that data as an argument.
- When this happens your callback function will run with the data passed into it as an argument meaning you are able to use that data for whatever you need it for.
So, simply put it is a way to request data and have it passed into a function of your choosing when it loads in.
It does this because the HTML script tag will evaluate the JSONP as JavaScript and try to run it.
What is JSONP meant to mitigate?
The reason why JSONP was created and what JSONP was meant to mitigate was to avoid issues with the same-origin policy where client-side applications couldn’t request resources from other origins outside of their own including data like JSON.
JSONP bypasses this because the HTML script tag is exempt from the same-origin policy meaning it could access scripts from other pages, origins and sites meaning if you needed to get data, the HTML script tag src attribute was one of the few ways in which you could do it.
The problem is that if you just load standard JSON into a script tag nothing will happen because you need to assign the JSON data to a variable that you can access to be able to use it.
This is where JSONP comes in and runs a callback function where the data then gets passed into it.
The problem with JSONP is that if the resource you are requesting wants to, it can inject malicious code into your site, or directly into your function which is not good.
Since all of this, we now have CORS(Cross-origin resource sharing) which means we have more control as to what resources we accept and reject on a given page which means we no longer need to rely on JSONP because we can accept raw JSON data instead.
To this day you can still use JSONP if you want to because it is essentially just loading in a JavaScript resource that will run a function and pass in data.
Because of CORS we can also use JSONP within applications that use React, or get JSONP data via ajax, fetch or axois but we would need to create our own parsing function or make use of a library such as jsonp.
If you do need to do this, it would still be worth considering using a script tag in these situations because it will save a lot of time, resources and hassle.
JSONP VS JSON
The best way to describe JSONP vs JSON is that JSON is raw data, and JSONP is JavaScript with the data embedded into it.
JSON (JavaScript Object Notation) is raw data that can be parsed and then used in JavaScript, whilst JSONP (JSON with Padding) is data that comes with a callback function. You create the function on your page/application, when the JSONP loads in it calls that function and passes in the data as an argument.
How to use JSONP (JSON-P) with JavaScript
Using JSONP with JavaScript is actually pretty easy to do, although it is going to be a mix of JavaScript and HTML because that was originally how it was intended to be used.
Here is an example of how to use JSONP in JavaScript:
<script>
window.jsonFlickrFeed = data => {
console.log(data)
}
</script>
<script
type="text/javascript"
src="https://www.flickr.com/services/feeds/photos_public.gne?format=json"
></script>
As you can see we first create a function that can handle the data. When we create this function the name has to match the function that is called from the jsonp data.
Usually most of these JSONP API’s will let you pass in the function name to the API, in the example above though it doesn’t and it will always call the function jsonFlickrFeed
which is why we have named our function that.
To check this, it is pretty easy to do, just open up the JSONP feed/API and look at the function it calls, it will be the very first thing in the data.

To check this, it is pretty easy to do, just open up the JSONP feed/API and look at the function it calls, it will be the very first thing in the data.
It will look something like this:
someFunctionBeingCalled({ hello: "World" })
So just make sure that your JavaScript function is called the same thing as what the JSONP data is calling, for example here it would be someFunctionBeingCalled
.
After we have our function it is just a case of loading in the data via a script element.
Once the data loads in, the JavaScript function will be executed which will then call your function.
Using the above example here is what the output looks like:
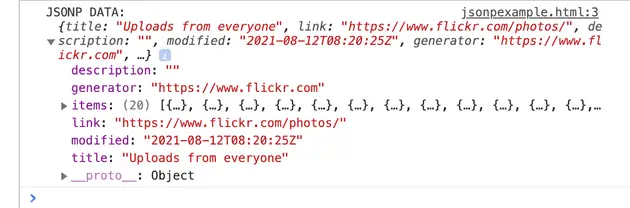
JSONP DATA:
{title: "Uploads from everyone", link: "https://www.flickr.com/photos/", description: "", modified: "2021-08-12T08:20:25Z", generator: "https://www.flickr.com", …}
description: ""
generator: "https://www.flickr.com"
items: (20) [{…}, {…}, {…}, {…}, {…}, {…}, {…}, {…}, {…}, {…}, {…}, {…}, {…}, {…}, {…}, {…}, {…}, {…}, {…}, {…}]
link: "https://www.flickr.com/photos/"
modified: "2021-08-12T08:20:25Z"
title: "Uploads from everyone"
Summary
There we have what is JSONP and How to use JSONP in JavaScript, if you want more like this be sure to check out some of my other posts!