Nullish Coalescing and Optional Chaining are two new features in JavaScript that were introduced in ECMAScript 2020, and in this post we are going to go over what they can do and how to use them.
If you are unfamiliar with what these two are you might recognize them in code form.
The Nullish Coalescing Operator looks like two question marks in JavaScript (??
), whilst the Optional Chaining Operator looks like a question mark and a dot/period in JavaScript (?.
).
What is nullish coalescing and optional chaining?
Nullish Coalescing is a way in which you use the Nullish Coalescing Operator in JavaScript to determine whether a value is nullish or not. The Optional Chaining Operator is there to safely call upon a variable or function without knowing if it exists or not.
Both of these operators make use of nullish values to determine what to do.
A nullish value in JavaScript is either null
or undefined
, you can read more about nullish values here.
The Nullish Coalescing Operator can best be thought of as a nullish version of the Logical OR Operator which checks if a value is falsy or truthy, whereas the Nullish Coalescing Operator checks if a value is nullish or not nullish.
Here is an example of the Nullish Coalescing Operator:
null ?? "Hello World" // "Hello World"
undefined ?? "Hello World" //"Hello World"
0 ?? "Hello World" // 0
And here is the same example with the Logical OR Operator:
null || "Hello World" // "Hello World"
undefined || "Hello World" // "Hello World"
0 || "Hello World" // "Hello World"
As you can see both the null and undefined yield the same result using the Logical OR Operator and the Nullish Coalescing Operator because they are both falsy and nullish values.
However, the result is different for the 0 because it is a falsy value but not a nullish value.
You can read into the Nullish Coalescing Operator in more detail by clicking here.
The Optional Chaining Operator can best be thought of as a safe version of the dot/period chaining operator (question mark dot, or ?.
).
We will go into more detail in the next section but very briefly it also checks for nullish values just like the Nullish Coalescing Operator and if it finds one it will return at that point instead of continuing down the chain.
For example here is how the Optional Chaining Operator looks:
const myObject = {
test: {
example: {
id: 123,
},
},
}
myObject.test.example.id // 123
myObject?.test?.example?.id // 123
myObject.notHere.example.id // Uncaught TypeError: Cannot read property 'example' of undefined
myObject?.notHere?.example?.id // undefined

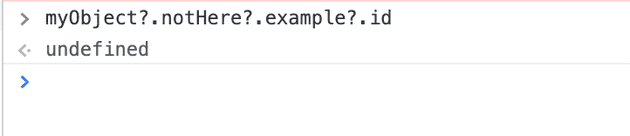
What is optional chaining and how can you use it?
Now we have covered both briefly, let’s take a look into what optional chaining is in a little more detail.
As we have said in the past section, the Optional Chaining Operator (question mark dot/period ?.
) does a nullish check on a value.
If that value is not nullish then it will continue onto the next chained value.
This is easy to think of in objects like in the previous example.
It provides a safe way of checking if properties exist or not. You might be wondering why this is useful if you already know what is in a property.
Well, sometimes we don’t always know what is in a property.
For example if we make a network request and we know that we have an optional field, one of the simplest ways to check for it is to use the Optional Chaining Operator.
In an ideal world, we would know exactly what should be in every response given a set of parameters instead of having optional values that may or may not be there, but for the most part this is not the case and we do have to deal with optional properties.
This becomes really obvious to see when you are using TypeScript with Optional Chaining as well.
On top of objects, we can also use the Optional Chaining Operator on arrays and functions as well!
Let’s take a look at how we can use optional chaining with arrays:
const myArray = [1, 2, 3]
myArray?.[0] // 1
myArray?.[2] // 3
myArray?.[3] // undefined
As you can see, instead of directly using an index, we only need to separate it with the Optional Chaining Operator to safely get the values in the array.
Just a quick note, whilst I say “safely”, it is important to remember to only use the Optional Chaining Operator if it is absolutely necessary.
If you know that a property exists, then you don’t need to use it.
Now let’s take a quick look at how the Optional Chaining Operator can be used with functions.
Let’s say you have an object that starts empty, as it gets passed around your application various properties get added and one may or may not be a function, and all you know is that it the function does exist then you need to call it, but you don’t want to throw an error if it does not exist.
Well, similar to the other examples we just need to call it with the Optional Chaining Operator.
const myObject = {
myNullFunction: null,
myFunction: () => "Hello World",
}
myObject?.myNullFunction?.() // undefined
myObject?.myFunction?.() // "Hello World"
What is the difference between nullish coalescing vs optional chaining?
The main difference between nullish coalescing vs optional chaining is that the Nullish Coalescing Operator cannot be used in chains of values and it is a logical operator that will return the left hand value if it passes, and it will return the right hand value if it fails, whereas the Optional Chaining Operator can be used in chains and will return undefined if the value you are attempting to find or call is nullish or it will move the chain to the next value in the chain that you are calling.
Here are both operators next to each other so you can see the difference:
const myObject = {
test: {
example: {
id: 123,
id2: null,
},
},
}
myObject.test.example.id2 ?? "Hello World" // "Hello World"
myObject?.test?.example?.id2 // null
myObject.test.example.doesNotExsit ?? "Hello World" // "Hello World"
myObject?.test?.example?.doesNotExist // undefined
myObject.test.test.doesNotExist ?? "Hello World" // Uncaught TypeError: Cannot read property 'doesNotExist' of undefined
myObject?.test?.test?.doesNotExist // undefined
And lastly, here is the two in combination to avoid getting an error like in the above example:
myObject?.test?.test?.doesNotExsit ?? "Hello World" // "Hello World"
Can I use optional chaining and nullish coalescing?
This has been around since ECMAScript 2020 and is supported in all major browsers, so as long as you are using the correct ecma script in your project and up to date browsers, the answer is most likely yes.
Checkout caniuse for a more detailed answer.
Summary
And there we have nullish coalescing (Two question marks ??
) and optional chaining (question mark dot/period ?.
)!
To summarise, the Optional Chaining Operator lets you perform a nullish check on chains, objects, arrays and functions which if it passes it will let you carry on the chain, otherwise it will return undefined.
And the Nullish Coalescing Operator will check a value to the left of it, and if it passes it will return that value, and if it fails it will return the value to the right hand side of it.