In this post we will be covering how to sort an array of objects by property value in JavaScript with as little technical jargon as possible so you will have everything you need right here without having to look any further!
Sorting an array of objects by property value in JavaScript is actually fairly straightforward thanks to the Array.prototype.sort method.
The Array.prototype.sort method accepts a callback function that runs to determine the order of each item in the array and that is what we are going to use to see how to sort an array of objects by a property value in JavaScript.
The sort method can be called on an array without providing a callback, which works fine for simple arrays such as an array of strings which it will sort alphabetically or numbers where it will sort it in numerical (kind of) order.
The reason why I say “Kind of” for sorting in numerical order is because it is not from the smallest to largest or largest to smallest, but instead by each character of a number.
Here is an example of this:
[1, 30, 4, 22, 21, 10000].sort() // [1, 10000, 21, 22, 30, 4]
Because of this it is always best to provide your own comparison callback function when using Array.prototype.sort so you don’t get any unexpected results.
The callback you pass into the sort method accepts two array items (arrayItemA
, and arrayItemB
), and needs to return an integer of either 0, greater than 0, or less than 0.
For simplicity here we will use -1
, 0
and 1
.
const sortCallback = (arrayItemA, arrayItemB) => { ... }
If you return -1
(or any integer less than 0) then arrayItemA will be sorted so it goes before arrayItemB.
If you return 0
then arrayItemA, and arrayItemB will not be sorted because they are the same value.
If you return 1
(or any integer greater than 0) then arrayItemA will be sorted so it goes after arrayItemB.
How to sort an array of objects by property value in JavaScript
To use Array.prototype.sort to sort an array of objects by property value in JavaScript, all we have to do is compare the property value in the sort callback and then return -1
, 0
, or 1
depending on how it compares to the other array item that we are comparing it against.
Let’s take a look at an example of how to sort an array of objects by property value in JavaScript:
const arrayOfObjects = [
{ apples: 5 },
{ apples: 2 },
{ apples: 30 },
{ apples: 1 },
]
const objectComparisonCallback = (arrayItemA, arrayItemB) => {
if (arrayItemA.apples < arrayItemB.apples) {
return -1
}
if (arrayItemA.apples > arrayItemB.apples) {
return 1
}
return 0
}
arrayOfObjects.sort(objectComparisonCallback) // [{ apples: 1 }, { apples: 2 }, { apples: 5 }, { apples: 30 }]
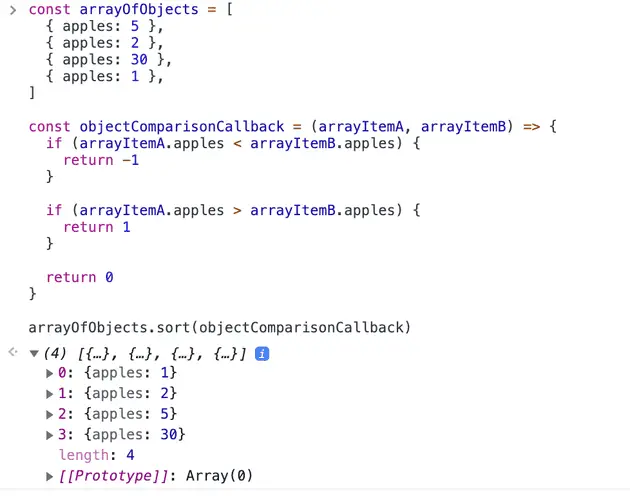
In this example we are using an array of objects each with a property called apples
, each property has a different number of apples
and we are sorting using this object property.
As you can see we are making use of the sort callback function to return -1
if the amount of apples in arrayItemB is greater than the amount of apples in arrayItemA, then we are doing the opposite of that when we return 1
and lastly, if neither of those conditions are triggered it means that the values are equal and then we return 0.
This then sorts the array in ascending order based on the values of the apples
property in our array of objects.
This same principle applies to any type of value in an array, even if you need to sort by multiple properties in an object.
You just need to always ensure your comparison callback function is working as expected.
So whether you have some key value pairs, dates, numbers or strings you can always use this method to sort and order your array of objects.
How to sort an array of objects by multiple properties in JavaScript
To sort an array of objects by multiple properties, is pretty much the same as sorting an array by a single property value, except you need to decide on the property hierarchy in the order.
Using our previous example, let’s once again have an array of objects containing apples
but this time we are going to add in a price
property, and we are going to want to sort this array first by price, and then by apples.
So this means that if we have an object with 2 apples
and a price of 5
, that should come before an object with 2 apples
and a price of 6
.
Let’s take a look at how this will look in JavaScript:
const arrayOfObjects = [
{ price: 5, apples: 4 },
{ price: 3, apples: 2 },
{ price: 5, apples: 3 },
{ price: 3, apples: 2 },
{ price: 3, apples: 3 },
]
const comparePrices = (arrayItemA, arrayItemB) => {
if (arrayItemA.price < arrayItemB.price) {
return -1
}
if (arrayItemA.price > arrayItemB.price) {
return 1
}
return 0
}
const compareApples = (arrayItemA, arrayItemB) => {
if (arrayItemA.apples < arrayItemB.apples) {
return -1
}
if (arrayItemA.apples > arrayItemB.apples) {
return 1
}
return 0
}
const objectComparisonCallback = (arrayItemA, arrayItemB) => {
// first sort by price
const priceOutcome = comparePrices(arrayItemA, arrayItemB)
if (priceOutcome !== 0) {
return priceOutcome
}
// at this point we will be looking at two equal prices so we now need to compare apples
return compareApples(arrayItemA, arrayItemB)
}
arrayOfObjects.sort(objectComparisonCallback)
// [
// { price: 3, apples: 2 },
// { price: 3, apples: 2 },
// { price: 3, apples: 3 },
// { price: 5, apples: 3 },
// { price: 5, apples: 4 },
// ]
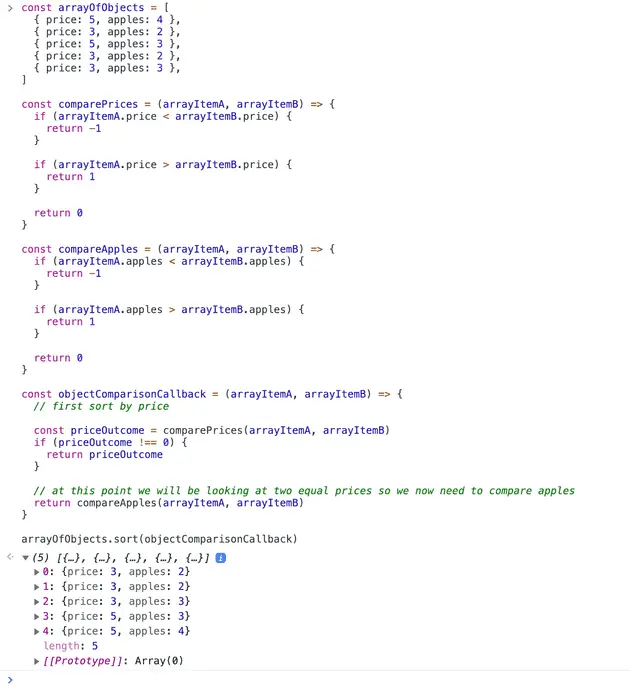
As you can see this is now sorting the array of objects using multiple properties.
First we are sorting by price, and then once we have the correct order of prices we are then sorting the apples within each price bracket to make an ascending order in our array.
The only other difference here is that the comparison callback has been split out into smaller helper functions to make everything easier to read and maintain.
Summary
There we have how to sort an array of objects by property value in JavaScript, if you want more like this be sure to check out some of my other posts!