In this post we will be covering how to rename a destructured variable in JavaScript with as little technical jargon as possible so you will have everything you need right here without having to look any further!
Often in JavaScript when you destructure a variable, argument, or prop you will need or want to rename it.
It might seem confusing at first, and a little tricky to remember which way round it works but all in all it is pretty easy to rename a destructured variable in JavaScript (destructuring assignment).
How to rename a destructured variable in JavaScript
Destructuring assignment or renaming a destructured variable in JavaScript is very similar to creating a field in an object, except instead of saving a variable to a field you are naming one of the fields from the object you are destructuring.
When renaming a destructured variable in JavaScript the left hand side will be the original field in the object, and the right hand side will be the name you provide to rename it to.
Here is an example:
const data = {
lt: 5,
lg: 10,
};
const { lt: lat, lg: long } = data;
console.log(lat, long); // 5, 10
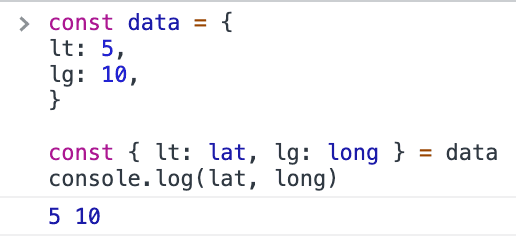
This comes in really handy if you need to destructure lots of similar objects, like request responses so that you can then name the responses exactly how you want to avoid confusion.
Another use case is within props(react) or arguments provided into functions.
Here is an example of rename destructured argument in JavaScript:
const someFunction = ({ message, previousMessage: lastMessage }) => {
console.log(lastMessage, message);
};
someFunction({ message: "world", previousMessage: "hello" }); // “hello world”

How to rename a destructured variable in JavaScript with a default value
Destructuring a variable in JavaScript with a default value can be done in the same way in which you would ordinarily do when looking at arguments in a function.
You will need to set the default using the assignment operator.
Here is an example of how to rename a destructured variable in JavaScript with a default value with an argument:
// javascript destructuring rename with default value in argument
const someFunction = ({
message,
previousMessage: lastMessage = "No previous message",
}) => {
console.log(lastMessage, message);
};
someFunction({ message: "" }); // "No previous message"
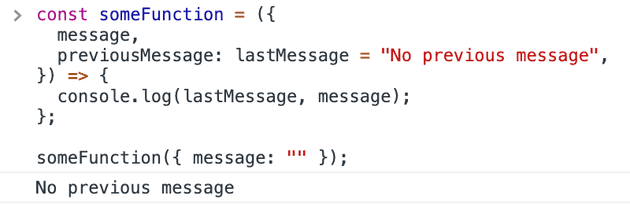
And here is an example with a standard variable:
// javascript destructuring rename with default value
const data = {};
const { lt: lat = 5, lg: long = 10 } = data;
console.log(lat, long); // 5, 10
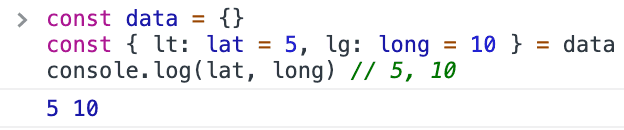
Summary
There we have how to rename a destructured variable in JavaScript, if you want more like this be sure to check out some of my other posts!