In this post we will be covering how to remove all spaces from a string in JavaScript with as little technical jargon as possible so you will have everything you need right here without having to look any further!
It is fairly common to need to remove all spaces from a string in JavaScript, just the same as it is common to replace spaces with other characters, or trim the string to remove spaces at the beginning and end.
There are a handful of ways in which we can do all of these things which will be covered in this post, but to start with we will look into how we can remove all spaces from a string in JavaScript in the simplest and easiest way that we can.
How to remove all spaces from a string in JavaScript
The easiest way to remove all spaces from a string in JavaScript is to make use of the String.prototype.replaceAll method to replace all space characters in a string with an empty string.
The String.prototype.replaceAll method accepts either a regex or a string, as well as the string you want to replace the original string with.
Seeing as regex is always a little tricky to remember, let’s start by just using a string to replace all the spaces in a string by using an empty string which will cause all the spaces to be removed.
Here is how we can use replaceAll to do this in JavaScript:
const exampleString = "Lorem ipsum dolor sit amet"
exampleString.replaceAll(" ", "") // "Loremipsumdolorsitamet"
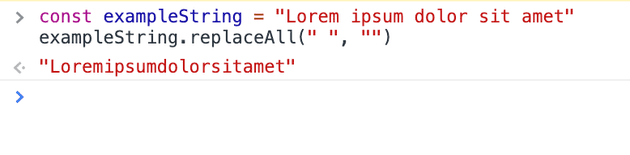
As you can see from the above example, we are using the replaceAll method to find all the space characters and replace them with no characters by providing the String.prototype.replaceAll method with an empty string.
By doing this we remove all the spaces in the string with ease.
It is worth noting that String.prototype.replaceAll will work in most modern browsers but it is not supported by internet explorer so you will need to use another solution if you need to support this browser which we will cover a little later on in this post.
Using regex to remove all spaces from a string in JavaScript
Using a regex to replace all the spaces from a string in JavaScript is also pretty straightforward, the only difference from using a string is that we need to remember the syntax for finding whitespaces with regex.
The regex we need to use to get all spaces in a string is /\s/g
.
Now to combine this with replaceAll we just need to add that into the first argument like so:
const exampleString = "Lorem ipsum dolor sit amet"
exampleString.replaceAll(/\s/g, "") // "Loremipsumdolorsitamet"

How to remove all spaces from a string in JavaScript without replaceAll (IE compatible)
To remove all spaces from a string in JavaScript without using the String.prototype.replaceAll method will mean we will now need to take a look at the String.prototype.split, and Array.prototype.join methods.
Using String.prototype.split and Array.prototype.join is a pretty well known way for removing certain characters like whitespace from a string in JavaScript, but now we have methods like String.prototype.replaceAll, we don’t really need to use the split/join way of doing it anymore.
However, if you need to support internet explorer then you might end up needing to use this method.
The String.prototype.split method accepts a string which it will use to split out your string into an array by finding all instances of the string you provide it.
When you do this, the split method will create an array of strings that contain all parts of the string except from the separator string that you used.
After you have split out your string into an array, you just need to join the array items back together to have a complete string with all the spaces (Or whichever separator you provided) removed.
Here is how this would look using our previous example:
const exampleString = "Lorem ipsum dolor sit amet"
exampleString.split(" ").join("") // "Loremipsumdolorsitamet"
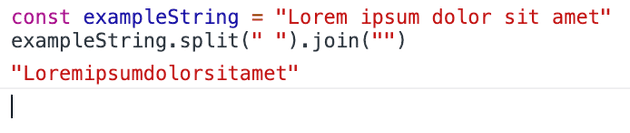
How to remove whitespace from beginning and end of a string in JavaScript
The last topic to cover in this post about removing spaces from a string in JavaScript is how to remove spaces specifically at the beginning or end of a string.
This process is called trimming a string and is used in many processes in many different languages.
In JavaScript we can trim the whitespace from the string just by calling the String.prototype.trim function which will do this for us!
Here is how it looks when using trim to remove the whitespace from the beginning and end of a string in JavaScript:
const exampleString = " Lorem ipsum dolor sit amet "
exampleString.trim() // "Lorem ipsum dolor sit amet"
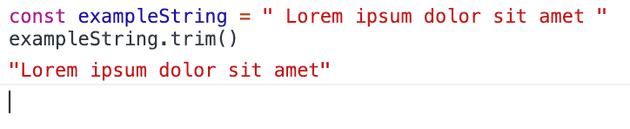
As you can see we have used the String.prototype.trim method on our string which has trimmed the whitespace from the beginning and end.
If you want to specifically only trim the beginning or the end of the string you can instead use one of the methods String.prototype.trimStart, or String.prototype.trimEnd.
Summary
There we have how to remove all spaces from a string in JavaScript, if you want more like this be sure to check out some of my other posts!