In this post we will be covering how to join two strings in JavaScript with as little technical jargon as possible so you will have everything you need right here without having to look any further!
There are many ways to join two strings in JavaScript. All of them are pretty easy to use, and we will go through each of them in this post.
How to join two strings in JavaScript
Currently one of the most popular methods to join two strings in JavaScript is by using template literals otherwise known as template strings.
Template literals involve the use of the back tick symbol ("``"
) to create the string and then breaking the template with a dollar symbol and curly braces to insert any variables you like.
Here is an example of using template literals in JavaScript to join two strings together:
// join two strings in javascript example
const stringOne = "Hello";
const stringTwo = "World";
const joinedString = `${stringOne} ${stringTwo}`;
console.log(joinedString); // "Hello World"
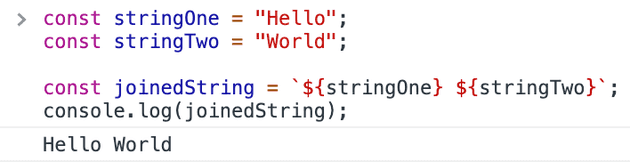
This is one of the most modern ways to join two strings in JavaScript and is usually preferred over concatenation.
Using concatenation to join two strings in JavaScript
To join to strings in JavaScript using concatenation all you need to do is make use of the addition operator.
String concatenation is a common way to join two strings together in not only JavaScript but many other programming languages as well.
Here is an example of using concatenation in JavaScript to join two strings:
const stringOne = "Hello";
const stringTwo = "World";
const joinedString = stringOne + " " + stringTwo;
console.log(joinedString); // "Hello World"
As well as simple usage of the addition operator, you can also mix it up a little because of how flexible it is.
Here is an example of concatenation with a string and a variable:
const stringOne = "Hello";
console.log(stringOne + " World"); // "Hello World"
Here is how to redefine a variable using the addition operator and concatenation:
let stringOne = "Hello";
stringOne += " World";
console.log(stringOne); // "Hello World"
A last option of concatenation is to call the function on a string instead of using the addition operator, however this is rarely used so I wouldn’t recommend it unless you have no other choice:
console.log("Hello".concat(" World")); // "Hello World"
How to join two strings in JavaScript in an Array
If you have two strings within an array, there is a simple way in which you can join them together, this also applies to other types as well so can be used for more than just strings.
The Array.prototype.join method allows you to join all elements of an array and convert it into a string, all you need to do is provide the character you want to join them together with.
Here is an example of how to join two strings in JavaScript in an Array with Array.prototype.join:
console.log(["Hello", "World"].join(" ")); // "Hello World"
Summary
There we have how to join two strings in JavaScript, if you want more like this be sure to check out some of my other posts!