In this post we will be covering how to get yesterday’s date in JavaScript with as little technical jargon as possible so you will have everything you need right here without having to look any further. It is actually very quick and easy to do and you won’t need to import any libraries!
How to get yesterday’s date in JavaScript
To get yesterday’s date in JavaScript all we need to do is make use of the Date Object’s methods Date.prototype.getDate, and Date.prototype.setDate in combination with the subtraction operator to subtract the date by 1.
In other words we first get today’s date, subtract one day from it, and then save the result in order to get yesterday’s date.
Here is an example of how to get yesterday’s date in JavaScript:
const today = new Date();
const yesterday = new Date();
yesterday.setDate(today.getDate() - 1);
console.log(yesterday); // Sun Oct 24 2021 22:22:07 GMT+0100 (British Summer Time)
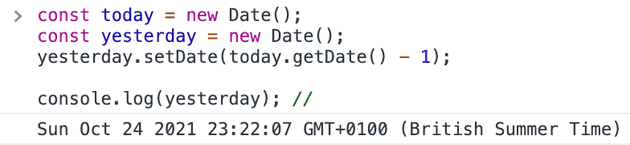
This will work for any day/date even if it is the first day of the month.
How to check if a date is today or yesterday in JavaScript
Now that we have the todays and yesterday’s date we can easily check if a given date is either today or yesterday.
Firstly we will need to reset the time so all the dates would be equal to the day and not the day and time.
After we reset the time we can then just perform a simple comparison to check if a date is today or yesterday.
const dateWithoutTime = someDate => new Date(someDate.toDateString());
const checkDateIsTodayOrYesterday = someDate => {
const today = dateWithoutTime(new Date());
const yesterday = dateWithoutTime(new Date());
yesterday.setDate(today.getDate() - 1);
const comparisonDateTime = dateWithoutTime(someDate).getTime();
if (
comparisonDateTime > today.getTime() ||
comparisonDateTime < yesterday.getTime()
) {
throw new Error("Invalid date - neither today or yesterday");
}
return comparisonDateTime === today.getTime() ? "today" : "yesterday";
};
const yesterdaysDate = new Date();
yesterdaysDate.setDate(yesterdaysDate.getDate() - 1);
console.log(checkDateIsTodayOrYesterday(new Date())); // 'today'
console.log(checkDateIsTodayOrYesterday(yesterdaysDate)); // 'yesterday'
console.log(checkDateIsTodayOrYesterday(new Date("01/02/2020"))); // Error "Invalid date - neither today or yesterday"
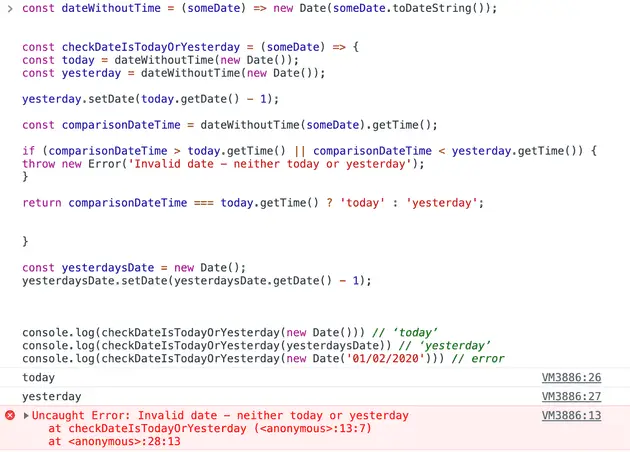
As you can see from the above example all we have done is package these principles into a couple of helper functions that can now check if a date that is provided is today or yesterday.
For more detail and information about comparing dates in JavaScript, have a read of my post about it here.
Formatting dates in JavaScript
When it comes to formatting dates in JavaScript the best option is to make use of a library such as date-fns to handle the formatting for you.
With date-fns, all you have to do is pass in your date and a string format and it will then do the rest of the work.
Here is a quick example of formatting yesterday’s date in JavaScript with date-fns to match yyyy-mm-dd or dd/mm/yyyy:
import { format } from "date-fns";
const yesterday = new Date();
yesterday.setDate(yesterday.getDate() - 1);
console.log(format(new Date(), "yyyy-MM-dd")); // 2021-10-24
console.log(format(new Date(), "dd/MM/yyyy")); // 24/10/2021
Summary
There we have how to get yesterday’s date in JavaScript, if you want more like this be sure to check out some of my other posts!