In this post we will be covering how to get the month name in JavaScript with as little technical jargon as possible so you will have everything you need right here without having to look any further!
To get the month name in JavaScript we have a few options, and in this post we will look at getting the month name from a JavaScript date, getting the month number from a JavaScript date using getMonth, and using date-fns to get the month name as well.
Let’s get started.
How to get the month name in JavaScript
The easiest way to get the month name in JavaScript from a date object is to make use of the Date.prototype.toLocaleString method which will provide us the date we need as a localised string.
To use this method all we need to do is call it on any JavaScript date object that we have initialized.
Once we have an initialized date object, all we need to do is pass in the locale, and the format that we want and it will do the rest.
Here is an example of how to use Date.prototype.toLocaleString to get the month name in JavaScript:
const xmas = new Date("12/25/2020")
xmas.toLocaleString("default", { month: "long" }) // December
The Date.prototype.toLocaleString uses internationalisation to provide the name of the month (or any other part of the date) to whichever locale you request.
In the above we used the default, which is going to be the best one to go with for most cases so that it is correct for the user, but we can also specifically set different locales.
Here is an example:
// US English
const xmas = new Date("12/25/2020")
xmas.toLocaleString("en-US", { month: "long" }) // December
// French
xmas.toLocaleString("fr", { month: "long" }) // décembre
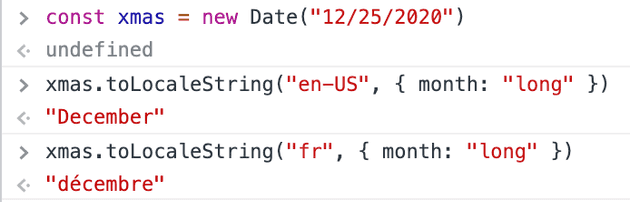
If you want to get the short version of the name of the month then you can instead pass in short
instead of long
.
Here is how the short name looks:
const xmas = new Date("12/25/2020")
xmas.toLocaleString("default", { month: short }) // Dec
Another way to make use of JavaScript internationalisation is to use the Intl.DateTimeFormat method which will effectively do the same thing as above.
Here is an example of how you can use Intl.DateTimeFormat
to get the month name in JavaScript:
const xmas = new Date('12/25/2020')
console.log(new Intl.DateTimeFormat(default, { month: 'long' }).format(xmas)) // December
How to use getMonth in JavaScript
Another option for finding the month name in JavaScript is to use the Date.prototype.getMonth method.
The getMonth method in JavaScript is a little confusing because it starts at 0
rather than 1
.
This means that January is month 0, and December is month 11.
So before you think that getMonth is wrong, it is actually correct, it just starts from 0 like the indexes of an array.
Here is an example of how you can use getMonth to get the month as a number in JavaScript:
const xmas = new Date("12/25/2020")
xmas.getMonth() // 11
If you want to get the month name by using this number you will need to perform a lookup on your own set of strings that use the month index as the position.
The best way to perform a lookup like this is with an ordered array of month names
For example you could do something like this:
const shortMonths = [
"Jan",
"Feb",
"Mar",
"Apr",
"May",
"Jun",
"Jul",
"Aug",
"Sep",
"Oct",
"Nov",
"Dec",
]
const xmas = new Date("12/25/2020")
shortMonths[xmas.getMonth()] // Dec
The above works because both the getMonth method and the array start at the 0th index which means it will directly translate into the correct index as long as the array is in the correct order.
How to get the name of the month with date-fns
To get the month name with date-fns in JavaScript we need to use the format method.
When we use the format method we just need to format the date string so that it only returns the month.
Here is an example of how to get the month name with date-fns using the format method:
import { format } from "date-fns"
const xmas = new Date("12/25/2020")
format(xmas, "LLLL") // December
Summary
There we have how to get the month name in JavaScript, if you want more like this be sure to check out some of my other posts!