In this post we will be covering how to export multiple functions in JavaScript with as little technical jargon as possible so you will have everything you need right here without having to look any further!
When it comes to exporting multiple functions in JavaScript, ES6 has made this really easy to do with modules.
In this post we are going to look at everything you need to know to be able to export multiple functions in a JavaScript file, and even multiple variables.
How to export multiple functions in JavaScript
To export multiple functions in JavaScript, the easiest way to do so is by using the export statement before each function you want to export. As long as you do not export functions as default then you can export as many functions as you like from a module.
All you have to do is apply the statement before each of your function definitions and as long as you are using JavaScript with ES6 or later which most modern projects will be, then the functions will be exported.
You can use the export statement as many times as you like in a single file.
Here is an example of how to export multiple functions in JavaScript:
export function exampleFunctionOne() {
console.log("Hello World")
}
export function exampleFunctionTwo() {
console.log("Exporting multiple functions from a module!")
}
export function exampleFunctionFour() {
console.log("JavaScript is awesome!")
}
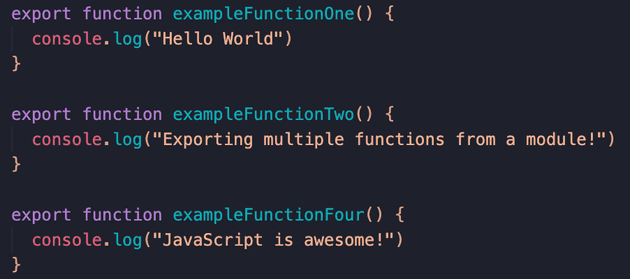
As you can see in this example exporting multiple functions with JavaScript is pretty easy, this type of exporting is known as named exports.
When it comes to importing these functions all you have to do is reference them as if the module is an object that you need to destructure.
Here is an example of how to import multiple functions in JavaScript from a single module:
import { exampleFunctionOne, exampleFunctionTwo } from "./some-js-file"
When you can’t export multiple functions in JavaScript
The only time you can’t export multiple functions in JavaScript from a module is if you are trying to export more than one default export.
You can only have one default export for any given module, but this does not mean you are then limited to only exporting one function, it simply means you can only export one default export.
As long as you only have one default export, you can still have as many named exports as you like.
Here is an example of exporting a default export with multiple named exports:
export default function myDefaultFunction() {}
export function namedExportExample() {}
export function namedExportExampleTwo() {}
And these can then be imported from the module into another like so:
import myDefaultFunction, {
namedExportExample,
namedExportExampleTwo,
} from "./some-js-file"
How to export multiple variables and arrow functions in JavaScript
Once again, exporting multiple variables in JavaScript is pretty easy and pretty similar to how we would export multiple functions from a module.
The only real difference is that we would be using a variable rather than a function.
Here is an example of how to export multiple variables and arrow functions in JavaScript:
export const hi = "Hello World"
export const myArrowFunction = (a, b) => a + b
And once again, this would be imported in exactly the same way as we import named functions.
Other ways to export multiple functions in JavaScript
Lastly, let’s take a look at a few more options for exporting multiple functions in JavaScript
We can export multiple functions as an object like so:
function example() {}
function exampleTwo() {}
export { example, exampleTwo }
If using ES6 or later is not an option we can use module.exports to be able to export functions instead:
function example() {}
function exampleTwo() {}
module.exports = {
example,
exampleTwo,
}
Summary
There we have how to export multiple functions in JavaScript, if you want more like this be sure to check out some of my other posts!