In this post we will be covering how to check if a string contains a substring in JavaScript with as little technical jargon as possible so you will have everything you need right here without having to look any further!
Sometimes you might need to check if a string contains a substring in JavaScript, and in this post we are going to look at just how to do that.
Before we get started it is important to just note that a JavaScript substring is simply a string within another string which you can think of as a set of characters.
For example, in the string "Hello World"
the word "Hello"
would be a substring, just like "World"
or even just a couple of characters like "lo Wo"
would also be a substring.
How to check if a string contains a substring in JavaScript (ES6)
To check if a string contains a substring in JavaScript the easiest way is to use String.prototype.includes which accepts a substring as an argument and will return a boolean value which will tell us if the string includes that substring or not.
This method (String.prototype.includes) is a modern approach (ES6 or newer).
Here is an example of how you can use it in JavaScript:
const stringExample = "Hello World"
const substringExample = "Hello"
stringExample.includes(substringExample) // true
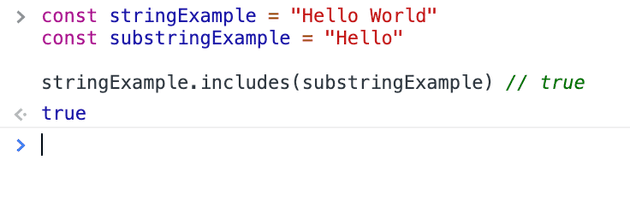
This will perform a case sensitive check, If you instead want a case insensitive check (so that upper and lowercase do not matter) then you will need to convert the string and substring to lower or uppercase first like so:
const stringExample = "Hello World"
const substringExample = "HeLLO"
stringExample.includes(substringExample) // false
stringExample.toLowerCase().includes(substringExample.toLowerCase()) // true

It is important to note that whilst String.prototype.includes is supported in almost all modern browsers it is not supported in internet explorer.
For most of us this won’t be an issue because most applications no longer support internet explorer either, however if you do need to support it then you would instead need to make use of a polyfill for String.prototype.includes or use String.prototype.indexOf.
Creating an easy polyfill for String.prototype.includes to check for a substring in JavaScript
If you need to add in a polyfill for String.prototype.includes to check if a string contains a substring in JavaScript you can add one in without needing an external library with something like the following code snippet:
if (!String.prototype.includes) {
String.prototype.includes = function includes(substring, position) {
if (typeof substring !== "string") {
throw new Error("substring must be a string")
}
return this.indexOf(substring, position || 0) > -1
}
}
How to check if a string contains a substring in JavaScript with indexOf
Using String.prototype.indexOf to check if a string contains a substring in JavaScript is pretty easy as well and will be very similar to our polyfill example above.
The String.prototype.indexOf method does a very similar thing as the includes method, but instead of returning a boolean it just returns an integer.
This integer returned from indexOf can either be -1
if it can’t find the substring, or it will return the position of the substring in the string which is why it is called indexOf.
This means to perform a boolean check we just need to make sure that the integer returned is greater than -1
or that it is not equal to -1
.
Here is an example of how to use the JavaScript String method of indexOf to check if a string contains a substring:
const stringExample = "Hello World"
const substringExample = "Hello"
stringExample.indexOf(substringExample) > -1 // true
Once again this performs a case sensitive check, here is how you can perform a case insensitive check with indexOf:
const stringExample = "Hello World"
const substringExample = "HeLLO"
stringExample.indexOf(substringExample) > -1 // false
stringExample.toLowerCase().indexOf(substringExample.toLowerCase()) > -1 // true

How does String.prototype.substring fit in?
Now that we have covered the two main ways of how to check if a string contains a substring in JavaScript, where does the method String.prototype.substring()
fit into this?
Well it doesn’t. String.prototype.substring is a method to get a substring from a string given the position rather than check if a substring is within a string.
That is why we can’t use it to check if a string contains another string/substring.
Summary
There we have how to check if a string contains a substring in JavaScript, if you want more like this be sure to check out some of my other posts!